springboot+mybatis如何执行存储过程,给一个详细的案例代码。并给注释
时间: 2024-04-16 08:28:48 浏览: 272
当使用Spring Boot和MyBatis执行存储过程时,你可以按照以下步骤进行操作:
1. 创建存储过程:首先,在数据库中创建存储过程。这里我们假设已经创建了一个名为`get_user`的存储过程,该存储过程接收一个用户ID作为输入参数,并返回该用户的信息。
```sql
CREATE PROCEDURE get_user
(IN user_id INT, OUT user_name VARCHAR(255), OUT user_age INT)
BEGIN
SELECT name, age INTO user_name, user_age FROM users WHERE id = user_id;
END
```
2. 创建实体类:在Java代码中创建一个与存储过程返回结果对应的实体类。
```java
public class User {
private String name;
private int age;
// 省略构造函数、getter和setter方法
}
```
3. 创建Mapper接口:创建一个Mapper接口,定义调用存储过程的方法。
```java
@Mapper
public interface UserMapper {
@Select("CALL get_user(#{userId, mode=IN}, #{userName, mode=OUT, jdbcType=VARCHAR}, #{userAge, mode=OUT, jdbcType=INTEGER})")
void getUser(@Param("userId") int userId, @Param("userName") String userName, @Param("userAge") int userAge);
}
```
4. 创建Service层:在Service层中调用Mapper接口的方法。
```java
@Service
public class UserService {
private final UserMapper userMapper;
public UserService(UserMapper userMapper) {
this.userMapper = userMapper;
}
public User getUser(int userId) {
String userName = null;
int userAge = 0;
userMapper.getUser(userId, userName, userAge);
return new User(userName, userAge);
}
}
```
5. 配置数据库连接:在`application.properties`或`application.yml`文件中配置数据库连接信息。
```yaml
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=root
spring.datasource.password=123456
```
6. 运行代码:使用Spring Boot启动应用程序,并调用UserService的方法来执行存储过程。
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
现在,你可以通过调用UserService的getUser方法来执行存储过程并获取结果。注意,存储过程的输出参数需要在调用过程中作为方法参数传入,并且需要使用`@Param`注解进行命名。
阅读全文
相关推荐
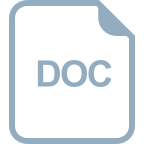
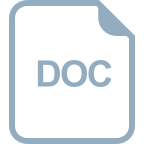
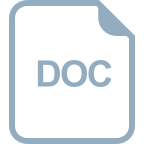
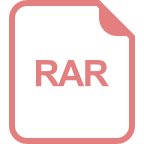
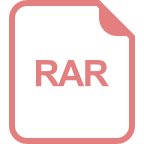
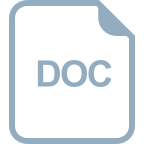
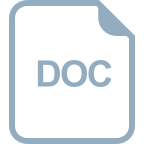
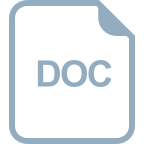
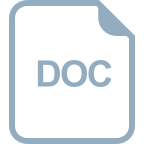
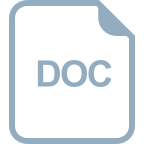
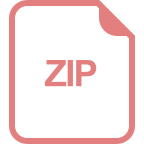
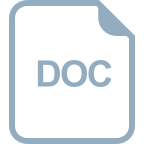
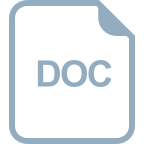
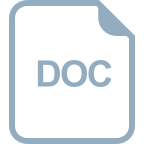
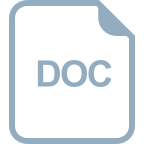
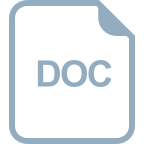
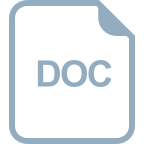
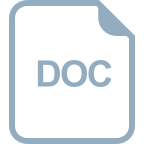
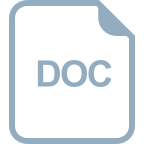