springboot debezium opengauss 如何实现捕获变更数据
时间: 2024-03-17 08:43:38 浏览: 177
要在Spring Boot应用程序中使用Debezium和OpenGauss来捕获变更数据,您需要执行以下步骤:
1. 在Spring Boot项目中添加Debezium和OpenGauss的依赖项。
2. 配置Debezium连接到OpenGauss数据库并启动Debezium引擎。
3. 创建一个Kafka生产者并将Debezium捕获的变更数据发送到Kafka主题。
4. 从Kafka主题中消费变更数据。
具体实现步骤如下:
1. 添加Debezium和OpenGauss的依赖项
在pom.xml中添加以下依赖项:
```
<dependency>
<groupId>io.debezium</groupId>
<artifactId>debezium-core</artifactId>
<version>1.3.0.Final</version>
</dependency>
<dependency>
<groupId>io.debezium.connector</groupId>
<artifactId>debezium-connector-opengauss</artifactId>
<version>1.3.0.Final</version>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.12</version>
</dependency>
<dependency>
<groupId>org.apache.kafka</groupId>
<artifactId>kafka-clients</artifactId>
<version>2.5.1</version>
</dependency>
```
2. 配置Debezium连接到OpenGauss数据库并启动Debezium引擎
在application.properties中添加以下配置:
```
# Debezium configuration
debezium.connector.name=opengauss
debezium.connector.class=io.debezium.connector.opengauss.OpenGaussConnector
debezium.offset.storage=kafka
debezium.offset.storage.topic=dbhistory.opengauss
debezium.offset.storage.partitions=1
debezium.offset.storage.replication.factor=1
debezium.snapshot.mode=when_needed
debezium.poll.interval.ms=5000
# OpenGauss configuration
database.hostname=localhost
database.port=5432
database.user=postgres
database.password=password
database.dbname=mydb
database.server.name=myserver
```
然后创建一个Debezium引擎实例,如下所示:
```
@Configuration
public class DebeziumEngineConfiguration {
@Value("${debezium.connector.name}")
private String connectorName;
@Value("${debezium.connector.class}")
private String connectorClass;
@Value("${debezium.offset.storage}")
private String offsetStorage;
@Value("${debezium.offset.storage.topic}")
private String offsetStorageTopic;
@Value("${debezium.offset.storage.partitions}")
private int offsetStoragePartitions;
@Value("${debezium.offset.storage.replication.factor}")
private short offsetStorageReplicationFactor;
@Value("${debezium.snapshot.mode}")
private String snapshotMode;
@Value("${debezium.poll.interval.ms}")
private long pollIntervalMs;
@Value("${database.hostname}")
private String hostname;
@Value("${database.port}")
private int port;
@Value("${database.user}")
private String user;
@Value("${database.password}")
private String password;
@Value("${database.dbname}")
private String dbname;
@Value("${database.server.name}")
private String serverName;
@Bean
public Configuration debeziumConfiguration() {
Configuration config = Configuration.create()
.with("connector.class", connectorClass)
.with("offset.storage", offsetStorage)
.with("offset.storage.topic", offsetStorageTopic)
.with("offset.storage.partitions", offsetStoragePartitions)
.with("offset.storage.replication.factor", offsetStorageReplicationFactor)
.with("name", connectorName)
.with("database.hostname", hostname)
.with("database.port", port)
.with("database.user", user)
.with("database.password", password)
.with("database.dbname", dbname)
.with("database.server.name", serverName)
.with("snapshot.mode", snapshotMode)
.with("poll.interval.ms", pollIntervalMs);
return config;
}
@Bean
public DebeziumEngine<ChangeEvent<String, String>> debeziumEngine() {
DebeziumEngine<ChangeEvent<String, String>> engine = DebeziumEngine.create(Json.class)
.using(debeziumConfiguration())
.notifying(this::handleEvent)
.build();
return engine;
}
private void handleEvent(ChangeEvent<String, String> event) {
// Handle event
}
}
```
3. 创建一个Kafka生产者并将Debezium捕获的变更数据发送到Kafka主题
首先创建一个Kafka生产者实例,然后在Debezium引擎中添加以下配置:
```
.with("database.history.kafka.bootstrap.servers", "${spring.kafka.bootstrap-servers}")
.with("database.history.kafka.topic", "dbhistory.opengauss")
```
然后在handleEvent()方法中将Debezium捕获的变更数据发送到Kafka主题,如下所示:
```
@Autowired
private KafkaTemplate<String, String> kafkaTemplate;
private void handleEvent(ChangeEvent<String, String> event) {
String key = event.key();
String value = event.value();
kafkaTemplate.send("mytopic", key, value);
}
```
4. 从Kafka主题中消费变更数据
创建一个Kafka消费者实例,然后从Kafka主题中消费变更数据,如下所示:
```
@KafkaListener(topics = "mytopic")
public void processMessage(ConsumerRecord<String, String> record) {
String key = record.key();
String value = record.value();
// Process message
}
```
阅读全文
相关推荐
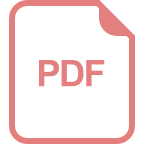
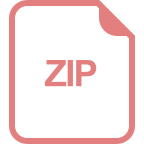

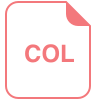



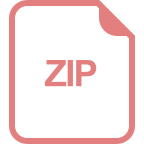
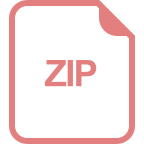
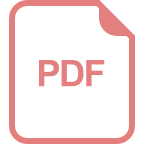
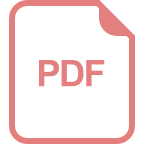
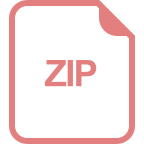
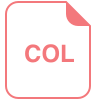
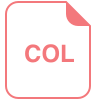
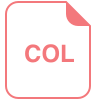
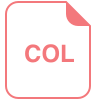
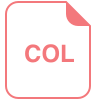
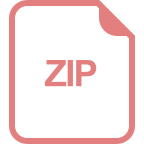