用vue和antd的评论组件写一个可以无限嵌套子评论的代码
时间: 2024-05-05 21:22:06 浏览: 12
以下是一个使用Vue和Antd的无限嵌套子评论的组件代码:
```html
<template>
<div class="comment-list">
<a-comment v-for="comment in comments" :key="comment.id">
<template #content>
<div>{{ comment.content }}</div>
<a-button type="link" @click="toggleReply(comment.id)">
{{ showReply(comment.id) ? '收起回复' : '回复' }}
</a-button>
<a-form-item v-if="showReply(comment.id)">
<a-input-text :value="replyContent[comment.id]" @input="updateReply(comment.id, $event.target.value)" />
<a-button type="primary" @click="addReply(comment.id)">回复</a-button>
</a-form-item>
<comment-list :comments="comment.children" />
</template>
</a-comment>
</div>
</template>
<script>
export default {
name: 'CommentList',
props: {
comments: {
type: Array,
default: () => []
}
},
data() {
return {
replyContent: {}
}
},
methods: {
toggleReply(commentId) {
this.replyContent[commentId] = ''
this.$set(this.replyContent, commentId, '')
this.$emit('toggle-reply', commentId)
},
showReply(commentId) {
return this.replyContent[commentId] !== undefined
},
updateReply(commentId, value) {
this.$set(this.replyContent, commentId, value)
},
addReply(commentId) {
const content = this.replyContent[commentId]
if (content) {
this.$emit('add-reply', commentId, content)
this.replyContent[commentId] = ''
this.$set(this.replyContent, commentId, '')
}
}
}
}
</script>
```
在上面的代码中,我们使用了Antd的`<a-comment>`和`<a-form-item>`组件来展示评论和回复表单。我们使用了Vue的`$set`方法来动态添加/删除`replyContent`对象中的属性,以确保子组件能够正确地更新。
我们还定义了一些方法来处理回复表单的显示/隐藏,回复内容的更新和添加新的回复。
在父组件中,我们可以使用以下方式来使用`<comment-list>`组件:
```html
<template>
<div class="comments">
<comment-list :comments="comments" @toggle-reply="toggleReply" @add-reply="addReply" />
</div>
</template>
<script>
import CommentList from './CommentList.vue'
export default {
name: 'Comments',
components: {
CommentList
},
data() {
return {
comments: [
{
id: 1,
content: '这是一条顶级评论',
children: [
{
id: 2,
content: '这是一条二级评论',
children: [
{
id: 3,
content: '这是一条三级评论'
}
]
},
{
id: 4,
content: '这是另一条二级评论'
}
]
}
]
}
},
methods: {
toggleReply(commentId) {
// 处理评论回复表单的显示/隐藏
},
addReply(commentId, content) {
// 处理添加回复的逻辑
}
}
}
</script>
```
在父组件中,我们通过传递一个名为`comments`的数组属性来渲染评论列表。我们还监听了`<comment-list>`组件的`toggle-reply`和`add-reply`事件来处理评论回复表单的显示/隐藏和添加回复的逻辑。
相关推荐
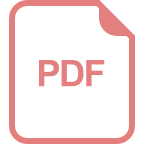
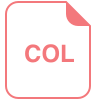
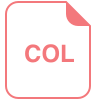
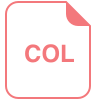
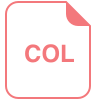
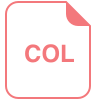









