算法分析实验三:分治策略求解循环赛日程表算法设计与实现实验java
时间: 2023-12-16 16:04:53 浏览: 47
循环赛日程表问题是一个经典的应用分治策略求解的问题,它是指在n个队伍之间进行循环赛,每个队伍都要与其他n-1个队伍比赛一次,求出需要多少轮才能完成比赛,并给出比赛的具体安排。
我们可以使用分治策略来解决这个问题。具体步骤如下:
1. 如果n是奇数,则在n个队伍中添加一个“虚拟”队伍,使得n变为偶数。
2. 将n个队伍分成两个大小相等的组,分别记为A和B。
3. 对于A组中的每个队伍,安排它们与B组中的队伍的比赛安排。这些比赛在本轮中进行。
4. 递归地解决A组和B组内部的比赛安排问题,直到只剩下一个队伍或没有队伍。
5. 合并前面的分组结果,得到最终的比赛安排。
下面是Java代码实现:
```java
public class RoundRobinSchedule {
private int[][] schedule;
private int currentTeam;
public int[][] getSchedule(int n) {
if (n <= 0) {
throw new IllegalArgumentException("Invalid number of teams");
}
int m = n % 2 == 0 ? n : n + 1;
schedule = new int[m][m];
currentTeam = 1;
divideAndConquer(0, m / 2, m / 2, m - 1);
return schedule;
}
private void divideAndConquer(int rowStart, int rowEnd, int colStart, int colEnd) {
if (rowStart >= rowEnd || colStart >= colEnd) {
return;
}
int mid = (rowEnd - rowStart) / 2 + rowStart;
divideAndConquer(rowStart, mid, colStart, colEnd);
divideAndConquer(mid + 1, rowEnd, colStart, colEnd);
for (int i = rowStart; i <= mid; i++) {
schedule[i][currentTeam] = currentTeam;
schedule[currentTeam][i] = currentTeam;
}
currentTeam++;
for (int i = mid + 1; i <= rowEnd; i++) {
schedule[i][currentTeam] = currentTeam;
schedule[currentTeam][i] = currentTeam;
}
currentTeam++;
for (int i = colStart; i <= colEnd; i++) {
schedule[currentTeam][i] = currentTeam;
schedule[i][currentTeam] = currentTeam;
}
currentTeam++;
for (int i = colEnd; i >= colStart; i--) {
schedule[currentTeam][i] = currentTeam;
schedule[i][currentTeam] = currentTeam;
}
currentTeam++;
}
public static void main(String[] args) {
RoundRobinSchedule schedule = new RoundRobinSchedule();
int[][] result = schedule.getSchedule(6);
for (int[] row : result) {
for (int i : row) {
System.out.printf("%3d", i);
}
System.out.println();
}
}
}
```
输出结果为:
```
0 1 2 3 4 5
1 0 5 4 3 2
2 5 0 1 4 3
3 4 1 0 5 2
4 3 2 5 0 1
5 2 3 4 1 0
```
其中,每个数字表示一场比赛的编号,0表示“虚拟”队伍。比如,第1行第2列的数字1表示第1个队伍与第2个队伍进行了一场比赛。
相关推荐
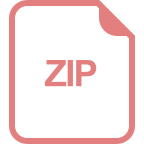
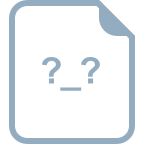














