换一种方法实现deleteItem(key) { let dataSourceLength = this.dataSource.length; this.dataSource = this.dataSource.filter(item => item.key !== key); if (dataSourceLength === 1) { this.addItem(0); } this.dataSource.forEach((item, index) => { item.key = index; }); this.$delete(this.editableData, key); },
时间: 2024-03-12 18:45:28 浏览: 22
这段代码的作用是从数据源 `dataSource` 中删除指定 `key` 的项,并且删除 `editableData` 对象中对应的属性。如果数据源中只剩下一项,那么会自动添加一项。
这里提供一种使用 `splice` 方法的实现方法:
```
deleteItem(key) {
let index = this.dataSource.findIndex(item => item.key === key);
if (index !== -1) {
this.dataSource.splice(index, 1);
this.dataSource.forEach((item, index) => {
item.key = index;
});
}
this.$delete(this.editableData, key);
if (this.dataSource.length === 1) {
this.addItem(0);
}
}
```
这个实现方法首先通过 `findIndex` 方法找到要删除的项在数据源中的索引,然后使用 `splice` 方法将其从数组中删除。接着,更新数据源中的 `key` 属性值,并且从 `editableData` 对象中删除对应的属性。最后,如果数据源中只剩下一项,自动添加一项。
相关问题
console.log(this.dataSource); for (let key in this.editableData) { const item = this.dataSource.filter((item) => key === item.key) if (!item) { throw new Error(`Object with key ${key} not found in this.dataSource`) } Object.assign(item, this.editableData[key]) this.$set(this.editableData, key, null) } // 删除 key 为 0 的键值对 delete this.editableData['0'] 这样保存成功但是没有更新编辑的数据
根据你提供的代码,我发现 `filter` 方法返回的是一个数组,而不是单个对象。因此,如果想要访问数组中的第一个对象,需要使用 `item[0]` 的方式进行访问。修改代码如下:
```
console.log(this.dataSource);
for (let key in this.editableData) {
const item = this.dataSource.filter((item) => key === item.key)[0]; // 修改这行代码
if (!item) {
throw new Error(`Object with key ${key} not found in this.dataSource`);
}
Object.assign(item, this.editableData[key]);
this.$set(this.editableData, key, null);
}
// 删除 key 为 0 的键值对
delete this.editableData['0'];
```
这段代码将 `item` 的取值改为 `this.dataSource.filter((item) => key === item.key)[0]`,也就是取数组中的第一个元素。这样就可以访问到数组中的对象了。
如果你仍然遇到问题,请提供更多的代码细节,以便我更好地帮助你解决问题。
saveAll() { for (let key in this.editableData) { const item = this.dataSource.filter((item) => key === item.key)[0]; if (!item) { throw new Error(`Object with key ${key} not found in this.dataSource`); } Object.assign(item, this.editableData[key]); this.$set(this.editableData, key, null); }}报错e.runtime.esm.js:619 [Vue warn]: Error in v-on handler: "Error: Object with key 0 not found in this.dataSource"
这个错误提示表示在 `this.dataSource` 数组中找不到 `key` 为 0 的对象,因此 `filter` 方法返回了一个空数组,导致访问了一个不存在的元素。
你可以检查一下 `this.editableData` 对象中是否包含了 `key` 为 0 的键值对,如果不存在,可以将其从 `this.editableData` 对象中删除,以避免再次访问时发生错误。
代码示例:
```
saveAll() {
for (let key in this.editableData) {
const item = this.dataSource.filter((item) => key === item.key)[0];
if (!item) {
throw new Error(`Object with key ${key} not found in this.dataSource`);
}
Object.assign(item, this.editableData[key]);
this.$set(this.editableData, key, null);
}
// 删除 key 为 0 的键值对
delete this.editableData['0'];
}
```
这段代码添加了一个 `delete` 语句,用于删除 `this.editableData` 对象中的 `key` 为 0 的键值对。这样,在下一次调用 `saveAll` 方法时,就不会再访问不存在的对象了。
相关推荐
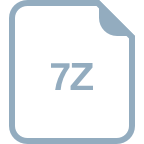
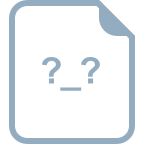
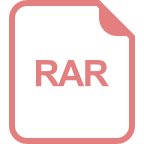











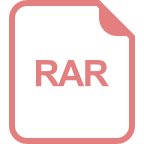
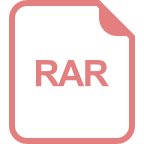