请给出详细示例,以java代码为例
时间: 2024-04-05 14:33:02 浏览: 98
好的,下面是一个简单的Java代码示例来生成ARQC:
```java
import javax.crypto.*;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.*;
import java.util.Arrays;
public class ARQCGenerator {
private static final String ARQC_ALGORITHM = "DESede/CBC/NoPadding";
private static final String DEK_ALGORITHM = "DESede";
public static void main(String[] args) throws Exception {
// 生成随机的DEK密钥
KeyGenerator keyGenerator = KeyGenerator.getInstance(DEK_ALGORITHM);
SecretKey dekKey = keyGenerator.generateKey();
// 生成随机的IV向量
SecureRandom random = SecureRandom.getInstanceStrong();
byte[] iv = new byte[8];
random.nextBytes(iv);
// 生成ARQC
String arqc = generateARQC("1234567890123456", 1000, dekKey, iv);
System.out.println("ARQC: " + arqc);
}
public static String generateARQC(String pan, int amount, SecretKey dekKey, byte[] iv) throws Exception {
// 计算ARQC的数据
byte[] data = calculateARQCData(pan, amount, iv);
// 加密数据得到ARQC
Cipher cipher = Cipher.getInstance(ARQC_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, dekKey, new IvParameterSpec(iv));
byte[] arqcBytes = cipher.doFinal(data);
// 将ARQC转换为十六进制字符串并返回
return bytesToHexString(arqcBytes);
}
private static byte[] calculateARQCData(String pan, int amount, byte[] iv) throws Exception {
// 计算ARQC的数据,包括PAN、交易金额和IV向量的哈希值
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] panBytes = pan.getBytes(StandardCharsets.UTF_8);
byte[] amountBytes = String.format("%012d", amount).getBytes(StandardCharsets.UTF_8);
byte[] data = Arrays.copyOf(panBytes, panBytes.length + amountBytes.length + iv.length);
System.arraycopy(amountBytes, 0, data, panBytes.length, amountBytes.length);
System.arraycopy(iv, 0, data, panBytes.length + amountBytes.length, iv.length);
byte[] hash = md.digest(data);
// 取哈希值的前8个字节作为ARQC的数据
return Arrays.copyOf(hash, 8);
}
private static String bytesToHexString(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02X", b));
}
return sb.toString();
}
}
```
以上代码演示了如何生成一个ARQC,其中用到了3DES加密算法和SHA-256哈希算法。注意,这只是一个简单的示例,实际使用中还需要考虑更多的安全因素。
阅读全文
相关推荐
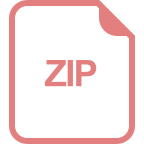
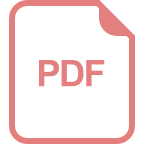
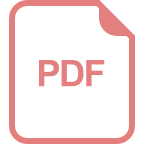















