python 写一个验证邮箱的正则表达式
时间: 2023-07-04 14:17:39 浏览: 108
可以使用以下正则表达式验证邮箱:
```python
import re
email = "example@example.com"
pattern = r"^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$"
if re.match(pattern, email):
print("Valid email address")
else:
print("Invalid email address")
```
解释一下这个正则表达式:
- `^` 匹配字符串的开始位置
- `[a-zA-Z0-9._%+-]+` 匹配至少一个字母、数字、下划线、百分号、加号、减号或点号
- `@` 匹配邮箱地址中的 @ 符号
- `[a-zA-Z0-9.-]+` 匹配至少一个字母、数字、点号或减号
- `\.` 匹配邮箱地址中的 . 符号(需要使用反斜杠转义)
- `[a-zA-Z]{2,}` 匹配至少两个字母,用于匹配邮箱地址中的顶级域名
- `$` 匹配字符串的结束位置
这个正则表达式可以匹配大部分常见的邮箱地址,但并不是完美的。在实际使用中,需要根据具体需求进行调整。
相关问题
邮箱验证python正则表达式
以下是一个使用正则表达式验证邮箱的Python示例:
```python
import re
def validate_email(email):
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
if re.match(pattern, email):
return True
else:
return False
email = "example@example.com"
if validate_email(email):
print("Valid email")
else:
print("Invalid email")
```
这个示例中,我们定义了一个`validate_email`函数,它接受一个邮箱地址作为参数。函数内部使用正则表达式模式来验证邮箱地址是否有效。如果邮箱地址有效,则返回True,否则返回False。
python实现邮箱正则表达式验证
可以使用Python的正则表达式库re来实现邮箱正则表达式验证。下面是一个示例代码:
```python
import re
def is_valid_email(email):
pattern = re.compile(r'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$')
return pattern.match(email)
email = 'example@gmail.com'
print(is_valid_email(email)) # True
```
这段代码使用了正则表达式`^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$`来匹配邮箱地址。
注意:这个表达式只能用来匹配一般的邮箱格式,不能匹配所有邮箱地址,如果有更严格的需求,需要自行修改正则表达式。
阅读全文
相关推荐
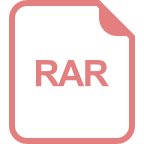
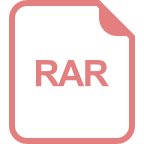
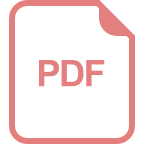








