Java中生成文件代码的测试类怎么写
时间: 2024-09-24 21:04:39 浏览: 70
在Java中,如果你有一个用于生成文件的工具类,比如`FileGenerator`,通常你会创建一个专门针对这个类进行单元测试的测试类,例如`FileGeneratorTest`。下面是一个简单的例子:
```java
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class FileGeneratorTest {
private final String expectedOutput = "This is the generated file content.";
@Test
public void testGenerateFile() {
// 创建一个FileGenerator实例
FileGenerator fileGenerator = new FileGenerator();
// 调用生成文件的方法并获取结果
String actualOutput = fileGenerator.generate("test.txt");
// 验证生成的内容是否符合预期
assertEquals(expectedOutput, actualOutput, "Generated file content should match expectation.");
// 检查文件是否存在
boolean fileExists = checkIfFileExists("test.txt");
assertTrue(fileExists, "Generated file should exist after calling generate method.");
// 清理资源,删除测试文件
deleteFileIfExists("test.txt");
// 验证文件是否已删除
assertFalse(fileExists, "Generated file should be deleted after test cleanup.");
}
// 辅助方法用于检查文件是否存在
private boolean checkIfFileExists(String fileName) {
return new File(fileName).exists();
}
// 辅助方法用于删除文件,如果存在的话
private void deleteFileIfExists(String fileName) {
if (new File(fileName).exists()) {
try {
new File(fileName).delete();
} catch (Exception e) {
throw new RuntimeException("Failed to delete temporary file", e);
}
}
}
}
```
在这个例子中,我们首先创建了`FileGenerator`的实例,然后调用了它的`generate`方法。接着,我们验证了生成的文件内容是否符合预期,并检查了文件是否确实存在于指定路径。最后,在测试结束后,我们删除了生成的文件以清理资源。
阅读全文
相关推荐




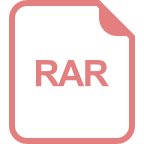












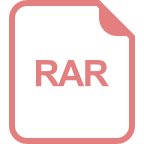
