pycharm编写程序,使一幅图像顺时针旋转90度再像x轴平移10像素,y轴平移20像素不改变图像大小
时间: 2024-03-14 11:47:10 浏览: 18
可以使用OpenCV库来实现这个功能。
首先,我们需要读取原始图像,可以使用OpenCV中的cv2.imread()函数实现。代码如下:
```python
import cv2
# 读取原始图像
img = cv2.imread('image.jpg')
```
接下来,我们需要将图像顺时针旋转90度。可以使用OpenCV中的cv2.rotate()函数实现。代码如下:
```python
# 将图像顺时针旋转90度
rotated_img = cv2.rotate(img, cv2.cv2.ROTATE_90_CLOCKWISE)
```
然后,我们需要将图像沿x轴平移10像素,y轴平移20像素。可以使用OpenCV中的cv2.warpAffine()函数实现。代码如下:
```python
# 定义平移矩阵
M = np.float32([[1, 0, 10], [0, 1, 20]])
# 将图像沿x轴平移10像素,y轴平移20像素
translated_img = cv2.warpAffine(rotated_img, M, (img.shape[1], img.shape[0]))
```
最后,我们可以将处理后的图像保存到本地。可以使用OpenCV中的cv2.imwrite()函数实现。代码如下:
```python
# 保存处理后的图像
cv2.imwrite('result.jpg', translated_img)
```
完整代码如下:
```python
import cv2
import numpy as np
# 读取原始图像
img = cv2.imread('image.jpg')
# 将图像顺时针旋转90度
rotated_img = cv2.rotate(img, cv2.cv2.ROTATE_90_CLOCKWISE)
# 定义平移矩阵
M = np.float32([[1, 0, 10], [0, 1, 20]])
# 将图像沿x轴平移10像素,y轴平移20像素
translated_img = cv2.warpAffine(rotated_img, M, (img.shape[1], img.shape[0]))
# 保存处理后的图像
cv2.imwrite('result.jpg', translated_img)
```
注意:在运行代码之前,需要确保安装了OpenCV库。可以使用以下命令来安装:
```bash
pip install opencv-python
```
相关推荐
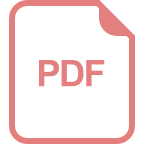
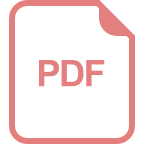














