MFC中矩形绕中心旋转是怎么计算旋转角度
时间: 2024-03-27 22:39:32 浏览: 165
在 MFC 中,如果要实现矩形绕中心旋转,需要计算旋转角度。
假设原始矩形的中心坐标为 (cx, cy),矩形的宽度为 w,高度为 h,旋转后的矩形中心坐标为 (cx', cy'),旋转角度为 angle。则有以下公式:
```
double angle = atan2(cy - cy', cx' - cx);
```
其中 atan2 函数是求取两个数的反正切值,可以返回一个弧度值,可以通过将其转换为角度值来得到最终的旋转角度。
需要注意的是,atan2 函数的第一个参数是 y 坐标的差值,第二个参数是 x 坐标的差值,这里的差值是旋转前后两个矩形中心坐标在 x 和 y 坐标上的差值。
最后,需要将角度值转换为弧度值,可以使用以下公式:
```
double radians = angle * PI / 180;
```
其中 PI 是一个常量,表示圆周率,可以使用 M_PI 来表示。这样就可以得到最终的旋转角度,可以通过旋转设备上下文来实现矩形的旋转。
相关问题
MFC中怎么在一个旋转后的矩形内绘制椭圆
要在一个旋转后的矩形内绘制椭圆,可以使用GDI+中的Graphics对象实现。具体步骤如下:
1. 创建一个GDI+的Graphics对象,将其与MFC的DC对象关联。可以使用Gdiplus::Graphics的构造函数实现,例如:
```c++
Gdiplus::Graphics graphics(pDC->GetSafeHdc());
```
2. 在绘制椭圆之前,先将Graphics对象的坐标系旋转一个角度,可以使用Gdiplus::Graphics的RotateTransform方法。例如,如果要旋转30度,可以使用以下代码:
```c++
graphics.RotateTransform(30);
```
3. 创建一个矩形,可以使用Gdiplus::RectF结构体实现,例如:
```c++
Gdiplus::RectF rect(100, 100, 200, 150); // 创建一个矩形
```
4. 在矩形内绘制椭圆,可以使用Gdiplus::Graphics的DrawEllipse方法和Gdiplus::GraphicsPath类实现。具体步骤如下:
1. 创建一个Gdiplus::GraphicsPath对象,表示椭圆的路径,例如:
```c++
Gdiplus::GraphicsPath path;
```
2. 将椭圆的路径添加到GraphicsPath对象中,可以使用Gdiplus::GraphicsPath的AddEllipse方法实现,例如:
```c++
path.AddEllipse(rect);
```
3. 将GraphicsPath对象的路径裁剪为矩形的路径,可以使用Gdiplus::Graphics的SetClip方法实现,例如:
```c++
graphics.SetClip(rect);
```
4. 绘制椭圆,可以使用Gdiplus::Graphics的FillPath方法和Gdiplus::SolidBrush类实现,例如:
```c++
Gdiplus::SolidBrush brush(Gdiplus::Color(255, 0, 0)); // 创建一个红色的画刷
graphics.FillPath(&brush, &path); // 填充椭圆
```
5. 销毁Graphics对象,以便后续的绘制不受影响。可以使用delete运算符,例如:
```c++
delete graphics;
```
完整的绘制代码如下:
```c++
void CMyView::OnDraw(CDC* pDC)
{
// 创建一个GDI+的Graphics对象
Gdiplus::Graphics graphics(pDC->GetSafeHdc());
// 将Graphics对象的坐标系旋转一个角度
graphics.RotateTransform(30);
// 创建一个矩形
Gdiplus::RectF rect(100, 100, 200, 150);
// 创建一个GraphicsPath对象,表示椭圆的路径
Gdiplus::GraphicsPath path;
path.AddEllipse(rect);
// 将GraphicsPath对象的路径裁剪为矩形的路径
graphics.SetClip(rect);
// 绘制椭圆
Gdiplus::SolidBrush brush(Gdiplus::Color(255, 0, 0));
graphics.FillPath(&brush, &path);
// 销毁Graphics对象
delete graphics;
}
```
这样就可以在一个旋转后的矩形内绘制椭圆了。
在MFC中,已知一个矩形在坐标系中间且四个顶点坐标确定,求它关于原点旋转45度后的顶点坐标的代码
在 MFC 中,可以利用 `CPoint` 类来表示点的坐标,利用 `CRect` 类来表示矩形。已知矩形的四个顶点坐标为 `(x1, y1)`, `(x2, y2)`, `(x3, y3)`, `(x4, y4)`,则可以利用以下代码计算出该矩形绕原点逆时针旋转45度后的四个顶点坐标:
```
#include <math.h>
CPoint RotatePoint(const CPoint& pt, double angle)
{
// 将点 pt 绕原点逆时针旋转 angle 度后的新坐标
double radian = angle * M_PI / 180.0;
int new_x = (int)(pt.x * cos(radian) - pt.y * sin(radian));
int new_y = (int)(pt.x * sin(radian) + pt.y * cos(radian));
return CPoint(new_x, new_y);
}
CRect RotateRect(const CRect& rect, double angle)
{
CPoint pt1(rect.left, rect.top);
CPoint pt2(rect.right, rect.top);
CPoint pt3(rect.right, rect.bottom);
CPoint pt4(rect.left, rect.bottom);
pt1 = RotatePoint(pt1, angle);
pt2 = RotatePoint(pt2, angle);
pt3 = RotatePoint(pt3, angle);
pt4 = RotatePoint(pt4, angle);
int left = min(min(pt1.x, pt2.x), min(pt3.x, pt4.x));
int top = min(min(pt1.y, pt2.y), min(pt3.y, pt4.y));
int right = max(max(pt1.x, pt2.x), max(pt3.x, pt4.x));
int bottom = max(max(pt1.y, pt2.y), max(pt3.y, pt4.y));
return CRect(left, top, right, bottom);
}
```
这里定义了两个函数:`RotatePoint` 和 `RotateRect`。`RotatePoint` 函数用于将点 `pt` 绕原点逆时针旋转指定的角度 `angle` 度,返回旋转后的新坐标。`RotateRect` 函数则利用 `RotatePoint` 函数来计算出矩形的四个顶点旋转后的坐标,再根据旋转后的四个顶点坐标来确定旋转后的矩形的位置和大小。最终,`RotateRect` 函数返回旋转后的矩形。
在调用 `RotateRect` 函数时,只需要将原始矩形作为参数传入即可。例如:
```
CRect rect(x1, y1, x4, y4);
CRect rotated_rect = RotateRect(rect, 45.0);
```
其中,`x1`、`y1`、`x4`、`y4` 分别表示原始矩形的左上角和右下角的坐标。`rotated_rect` 即为旋转后的矩形。
阅读全文
相关推荐
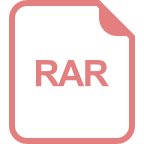
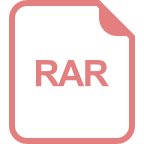
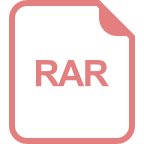
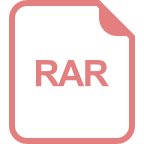
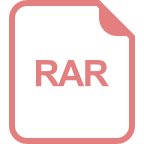
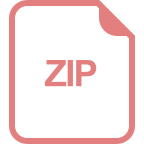
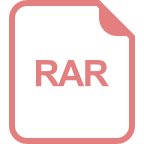
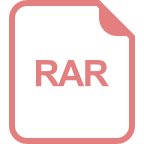
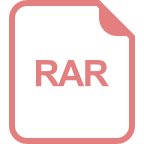
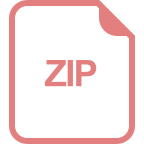
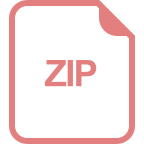
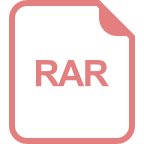
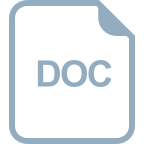

