编写三个不同的程序:cmd1.c, cmd2.c, cmd3.c,每个程序输出一句话,分别编译成可执行文件cmd1, cmd2, cmd3。然后再编写一个程序,模拟shell程序的功能,能根据用户输入的字符串(表示相应的命令名),去为相应的命令创建子进程并让它去执行相应的程序,而父进程则等待子进程的结束,然后再等待接收下一条命令。如果接收到的命令为exit,则父进程结束,如果接收到无效命令,则显示”command not found”,继续等待。
时间: 2024-02-22 12:56:38 浏览: 23
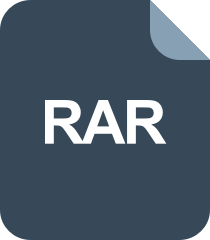
模拟的shell程序
以下是三个程序的代码:
cmd1.c:
```
#include <stdio.h>
int main() {
printf("This is command 1.\n");
return 0;
}
```
cmd2.c:
```
#include <stdio.h>
int main() {
printf("This is command 2.\n");
return 0;
}
```
cmd3.c:
```
#include <stdio.h>
int main() {
printf("This is command 3.\n");
return 0;
}
```
然后,我们编写一个模拟shell程序的代码,如下所示:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
char line[1024];
while (1) {
printf("> ");
if (!fgets(line, 1024, stdin)) break;
if (strcmp(line, "exit\n") == 0) {
break;
}
line[strlen(line) - 1] = '\0';
pid_t pid = fork();
if (pid == -1) {
printf("Failed to fork.\n");
return 1;
} else if (pid == 0) {
char *args[] = {line, NULL};
execvp(args[0], args);
printf("Command not found.\n");
return 1;
} else {
int status;
waitpid(pid, &status, 0);
}
}
return 0;
}
```
该程序通过循环不断等待用户输入命令,如果命令为exit,则退出程序。否则,该程序创建一个子进程,并使用execvp函数执行对应的命令程序。如果执行失败,则显示"command not found",否则父进程等待子进程结束后再继续循环等待用户输入。
阅读全文
相关推荐
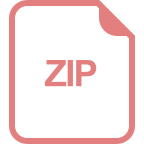
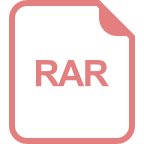
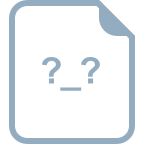
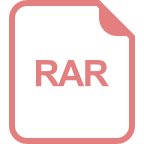
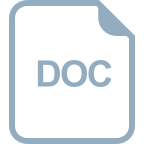
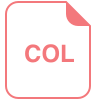
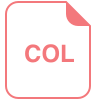



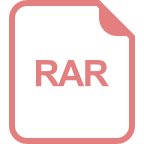
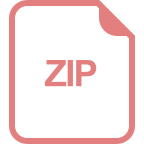
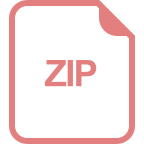
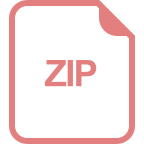
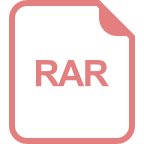
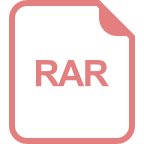