请你利用C++代码实现一下功能:处理一个csv文件,利用外排序进行排序。其中csv文件是string类型
时间: 2024-03-13 08:47:53 浏览: 26
好的,下面是利用C++代码实现csv文件的外排序:
```c++
#include <fstream>
#include <iostream>
#include <algorithm>
#include <string>
#include <vector>
#include <queue>
using namespace std;
const int MAX_SIZE = 1000000;
void merge(ifstream &file1, ifstream &file2, ofstream &out) {
string line1, line2;
getline(file1, line1);
getline(file2, line2);
while (!file1.eof() || !file2.eof()) {
if (line1.empty()) {
out << line2 << endl;
getline(file2, line2);
} else if (line2.empty()) {
out << line1 << endl;
getline(file1, line1);
} else {
if (line1 <= line2) {
out << line1 << endl;
getline(file1, line1);
} else {
out << line2 << endl;
getline(file2, line2);
}
}
}
}
void externalSort(string input_file, string output_file) {
ifstream input(input_file);
if (!input) {
cout << "Cannot open input file.\n";
return;
}
vector<string> buffer;
buffer.reserve(MAX_SIZE);
int file_index = 0;
while (!input.eof()) {
buffer.clear();
buffer.reserve(MAX_SIZE);
for (int i = 0; i < MAX_SIZE && !input.eof(); i++) {
string line;
getline(input, line);
buffer.push_back(line);
}
sort(buffer.begin(), buffer.end());
string file_name = "temp_" + to_string(file_index++);
ofstream output(file_name);
for (const auto &line : buffer) {
output << line << endl;
}
}
input.close();
priority_queue<pair<string, int>, vector<pair<string, int>>, greater<pair<string, int>>> pq;
for (int i = 0; i < file_index; i++) {
string file_name = "temp_" + to_string(i);
ifstream input(file_name);
if (input) {
string line;
getline(input, line);
pq.push(make_pair(line, i));
}
}
ofstream output(output_file);
while (!pq.empty()) {
auto top = pq.top();
pq.pop();
output << top.first << endl;
string file_name = "temp_" + to_string(top.second);
ifstream input(file_name);
if (input) {
string line;
getline(input, line);
if (!line.empty()) {
pq.push(make_pair(line, top.second));
}
}
}
output.close();
for (int i = 0; i < file_index; i++) {
string file_name = "temp_" + to_string(i);
remove(file_name.c_str());
}
}
int main() {
string input_file = "input.csv";
string output_file = "output.csv";
externalSort(input_file, output_file);
return 0;
}
```
这段代码的主要思路是将csv文件分割成多个小文件进行排序,然后再利用归并排序的思想进行合并。具体的实现过程如下:
1. 读取csv文件,将其中的内容分割成多个buffer,每个buffer包含最多MAX_SIZE个记录。
2. 对每个buffer进行内部排序,得到多个有序的小文件。
3. 利用优先队列,将所有小文件的第一条记录放入队列中,每次取出队列中最小的记录,将其写入输出文件中。同时,将对应小文件的下一条记录加入队列中,直到队列为空。
这样就可以利用外排序对csv文件进行排序了。
相关推荐
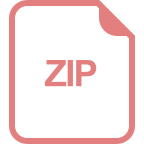
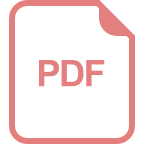
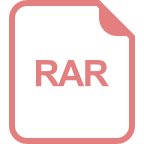
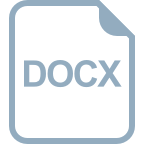
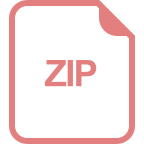
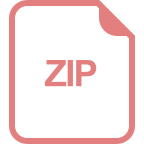
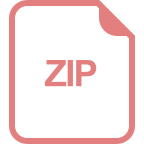
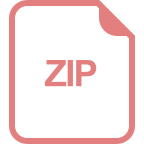
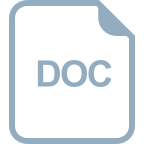
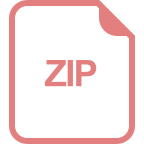
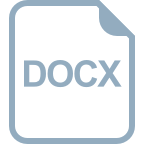
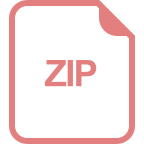
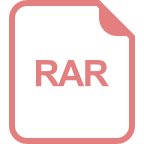
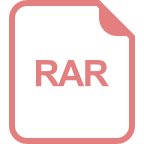