帮我用cpp写一份棋盘覆盖问题的代码
时间: 2024-10-13 07:05:50 浏览: 27
在C++中,我们可以使用深度优先搜索(DFS)或广度优先搜索(BFS)算法来解决经典的棋盘覆盖问题。这里我给出一个简单的8x8格子的例子,其中我们要放置骑士(Knight)来覆盖所有的格子。骑士可以跳跃两步并旋转90度,这构成了一个L形移动。
首先,我们创建一个二维数组表示棋盘,然后定义一个骑士类`Knight`,用于存储当前位置。接下来是一个函数`isSafeToMove`检查某个位置是否合法,最后主函数`solveChessboardCover`通过回溯法尝试所有可能的骑士初始位置。
```cpp
#include <vector>
#include <iostream>
class Knight {
private:
std::pair<int, int> position;
public:
Knight(int x, int y) : position{x, y} {}
bool isSafeToMove(const Knight &other, const std::vector<std::vector<int>>& board) {
int dx[] = {2, 1, -1, -2, -2, -1, 1, 2};
int dy[] = {1, 2, 2, 1, -1, -2, -2, -1};
for (int i = 0; i < 8; ++i) {
int newX = position.first + dx[i];
int newY = position.second + dy[i];
if (newX >= 0 && newX < 8 && newY >= 0 && newY < 8 && !board[newX][newY]) {
return true;
}
}
return false;
}
void move(Knight &other, std::vector<std::vector<int>>& board) {
int newX = other.position.first;
int newY = other.position.second;
board[position.first][position.second] = 1;
position = {newX, newY};
other.position = position;
}
};
bool solveChessboardCover(std::vector<std::vector<int>>& board, int startX, int startY) {
Knight knight(startX, startY);
board[startX][startY] = 1;
for (int i = 0; i < 8; ++i) {
for (int j = 0; j < 8; ++j) {
if (!board[i][j] && knight.isSafeToMove(knight, board)) {
knight.move(knight, board);
if (solveChessboardCover(board, i, j))
return true;
knight.move(knight, board); // 回溯
}
}
}
return false;
}
int main() {
std::vector<std::vector<int>> chessboard(8, std::vector<int>(8, 0));
if (solveChessboardCover(chessboard, 0, 0)) {
for (const auto &row : chessboard)
for (int cell : row)
std::cout << cell << " ";
std::cout << "\nBoard covered by a knight.\n";
} else {
std::cout << "No solution found.\n";
}
return 0;
}
```
这个程序会打印出一个由1表示骑士位置、0表示未覆盖的格子的棋盘。如果找不到解,则会输出"No solution found."。
阅读全文
相关推荐
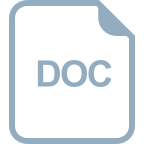
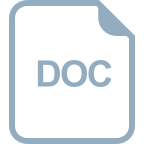
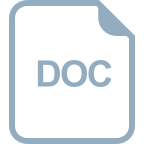
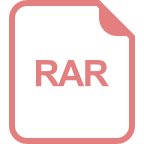
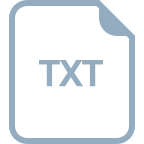
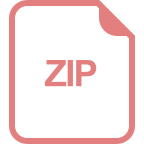
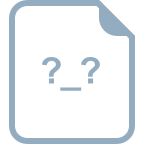
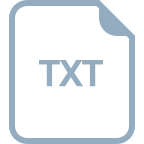
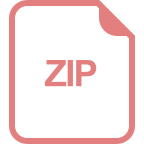
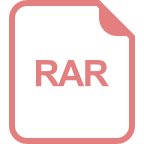
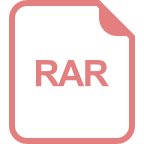
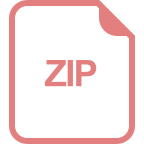
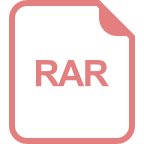