OpenCV 轮廓重心 QT
时间: 2024-12-26 09:19:06 浏览: 18
### 如何在 QT 中使用 OpenCV 计算图像轮廓的重心
为了实现这一目标,可以按照如下方法编写代码:
首先加载灰度图并转换为二值化图像以便更好地识别轮廓[^1]。
```cpp
#include <opencv2/opencv.hpp>
#include <QApplication>
int main(int argc, char **argv){
QApplication app(argc, argv);
// 加载原始灰度图像
cv::Mat src = cv::imread("path_to_image", cv::IMREAD_GRAYSCALE);
if(src.empty()){
qDebug() << "Could not open or find the image";
return -1;
}
// 创建窗口显示原图
cv::namedWindow("Original Image");
cv::imshow("Original Image", src);
// 将图像转为二值图像
cv::Mat binary;
cv::threshold(src, binary, 128, 255, cv::THRESH_BINARY | cv::THRESH_OTSU);
```
接着寻找所有轮廓,并计算这些轮廓各自的矩形边界以及质心坐标[^2]。
```cpp
std::vector<std::vector<cv::Point>> contours;
std::vector<cv::Vec4i> hierarchy;
// 查找轮廓
cv::findContours(binary.clone(), contours, hierarchy, CV_RETR_EXTERNAL, CV_CHAIN_APPROX_SIMPLE);
for (size_t i = 0; i < contours.size(); ++i) {
// 获取轮廓最小包围盒
cv::Rect boundingBox = cv::boundingRect(contours[i]);
// 绘制轮廓及其外接矩形到源图像上
cv::drawContours(src, contours, static_cast<int>(i), cv::Scalar(255));
rectangle(src, boundingBox.tl(), boundingBox.br(), cv::Scalar(255));
// 计算轮廓的几何矩来找到质心
cv::Moments M = moments(contours[i]);
int cx = M.m10 / M.m00;
int cy = M.m01 / M.m00;
// 在图像中标记质心位置
circle(src, cv::Point(cx,cy), 3, cv::Scalar(255), -1);
}
}
```
最后展示处理后的图像,在该图像中可以看到标记出来的各个轮廓及其中心点的位置。通过上述过程可以在 Qt 应用程序环境中利用 OpenCV 完成对输入图片内物体轮廓检测和重心定位的任务。
阅读全文
相关推荐


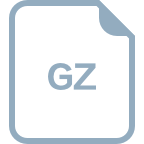




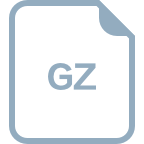



