短作业优先调度算法功能实现
时间: 2023-10-12 20:05:09 浏览: 82
短作业优先调度算法(Shortest Job First Scheduling Algorithm, SJF)是一种非抢占式的进程调度算法。它是按照作业的执行时间或者所需的CPU时间进行排序,并按照排序结果优先选择执行时间最短的作业。
SJF算法的实现步骤如下:
1. 获取所有进程的执行时间或所需CPU时间,并按照执行时间升序排序。
2. 将排序后的进程按顺序加入就绪队列中。
3. 从就绪队列中选择执行时间最短的进程,分配CPU资源给该进程进行执行。
4. 执行完毕后,将该进程从就绪队列中移除,并将执行结果输出。
5. 重复执行3、4步骤,直到所有进程都执行完毕。
以下是一个简单的SJF算法的实现代码,其中,Process类表示进程,包含进程ID和执行时间两个属性,ProcessList类表示进程列表,包含添加进程、按执行时间排序和获取下一个进程等方法,SJFScheduler类表示SJF调度器,包含调度方法schedule和结果输出方法printResult:
```python
class Process:
def __init__(self, pid, execute_time):
self.pid = pid
self.execute_time = execute_time
class ProcessList:
def __init__(self):
self.process_list = []
def addProcess(self, process):
self.process_list.append(process)
def sortByExecuteTime(self):
self.process_list.sort(key=lambda x: x.execute_time)
def getNextProcess(self):
if len(self.process_list) > 0:
return self.process_list.pop(0)
else:
return None
class SJFScheduler:
def __init__(self, process_list):
self.process_list = process_list
self.ready_queue = ProcessList()
self.time = 0
def schedule(self):
self.ready_queue.addProcess(self.process_list.getNextProcess())
while True:
current_process = self.ready_queue.getNextProcess()
if current_process is None:
break
print("Process", current_process.pid, "is executing")
self.time += current_process.execute_time
print("All processes are executed in", self.time, "units of time")
def printResult(self):
print("Process execution order:")
for process in self.process_list.process_list:
print(process.pid)
```
可以通过以下代码创建一个进程列表,并通过SJFScheduler类进行调度:
```python
process_list = ProcessList()
process_list.addProcess(Process(1, 5))
process_list.addProcess(Process(2, 3))
process_list.addProcess(Process(3, 8))
process_list.addProcess(Process(4, 2))
scheduler = SJFScheduler(process_list)
scheduler.schedule()
scheduler.printResult()
```
输出结果为:
```
Process 4 is executing
Process 2 is executing
Process 1 is executing
Process 3 is executing
All processes are executed in 18 units of time
Process execution order:
1
2
3
4
```
阅读全文
相关推荐
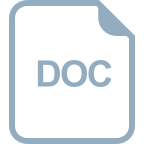
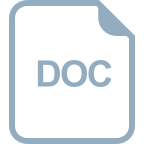
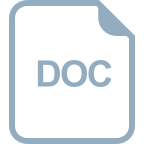
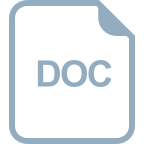
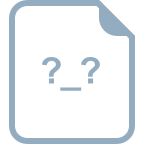
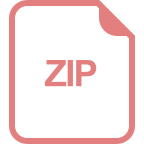
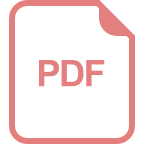
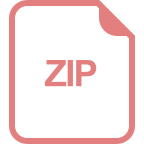
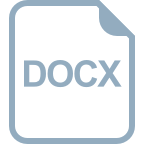
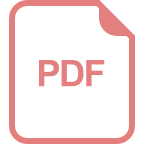
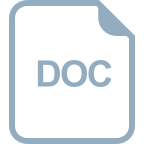
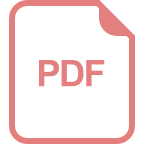
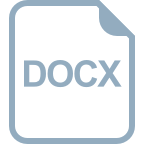
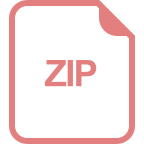
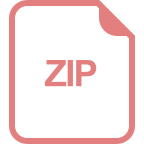
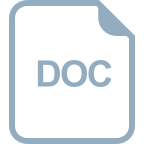
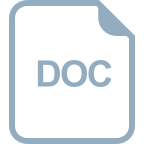