python selenium 鼠标移动
时间: 2023-06-05 17:47:51 浏览: 220
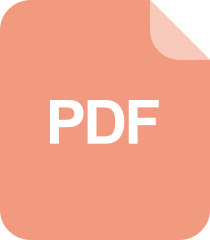
玩转python selenium鼠标键盘操作(ActionChains)
使用Python Selenium可以通过以下方式移动鼠标:
1. 使用ActionChains类中的move_to_element()方法将鼠标移动到指定元素上。
例如:
```python
from selenium.webdriver import ActionChains
# 定位到需要移动到的元素
element = driver.find_element_by_id("element_id")
# 创建ActionChains对象
actions = ActionChains(driver)
# 将鼠标移动到指定元素上
actions.move_to_element(element).perform()
```
2. 使用ActionChains类中的move_by_offset()方法将鼠标相对于当前位置移动指定的像素数。
例如:
```python
from selenium.webdriver import ActionChains
# 创建ActionChains对象
actions = ActionChains(driver)
# 将鼠标向右移动100像素,向下移动50像素
actions.move_by_offset(100, 50).perform()
```
以上是Python Selenium中移动鼠标的两种方式,可以根据实际需求选择使用。
阅读全文
相关推荐
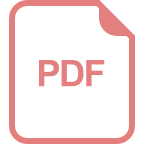
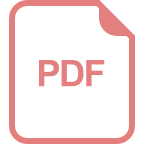















