用c++写代码创建一个结构体ST,成员有num(学号),name(姓名),score(成绩),从键盘输入N个人员信息,按score(成绩)降序输出每个学生的记录,在main函数中输入数据,在另一个函数中排序并输出。
时间: 2023-06-13 09:03:33 浏览: 99
下面是使用C语言编写的程序,实现创建一个结构体ST,输入N个人员信息,按照score(成绩)降序输出每个学生的记录:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct ST {
int num; // 学号
char name[20]; // 姓名
int score; // 成绩
};
void sort(struct ST *p, int n);
int main() {
int n;
printf("请输入学生数量:");
scanf("%d", &n);
struct ST *students = (struct ST *) malloc(n * sizeof(struct ST));
for (int i = 0; i < n; i++) {
printf("请输入第%d个学生的学号、姓名和成绩(用空格分隔):", i + 1);
scanf("%d %s %d", &students[i].num, students[i].name, &students[i].score);
}
sort(students, n);
printf("\n按成绩降序输出每个学生的记录:\n");
for (int i = 0; i < n; i++) {
printf("学号:%d\t姓名:%s\t成绩:%d\n", students[i].num, students[i].name, students[i].score);
}
free(students);
return 0;
}
void sort(struct ST *p, int n) {
struct ST temp;
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (p[i].score < p[j].score) {
temp = p[i];
p[i] = p[j];
p[j] = temp;
}
}
}
}
```
程序中定义了一个结构体ST,包含学号num、姓名name和成绩score三个成员变量。sort函数用于对学生信息进行排序,按照成绩降序排列。在main函数中,先读入学生数量n,然后动态分配n个结构体变量的内存,依次读入每个学生的信息,最后调用sort函数进行排序,输出每个学生的记录。
阅读全文
相关推荐














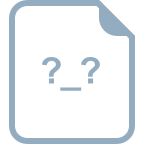