用eclipse写使用随机函数生成四则运算的出题界面,用户可以对出题给出答案程序能够自动判断对错,先期设定运算题型,数据大小,题目数量,最后根据批改给出测试成绩的代码
时间: 2024-02-24 10:56:14 浏览: 104
好的,以下是使用Java语言在Eclipse中编写的生成四则运算题目、用户答题、自动批改的代码示例。代码注释中有详细的说明。注意:本代码仅作为示例,具体实现方式可能与实际需求有所不同。
```
import java.util.Random;
import java.util.Scanner;
public class FourOperationsQuiz {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Random random = new Random();
// 设定题目类型和数据大小
int maxNum = 100; // 数据范围
String[] operators = {"+", "-", "*", "/"}; // 运算符
// 输入题目数量
System.out.print("请输入题目数量:");
int questionNum = sc.nextInt();
// 生成题目和答案
String[] questions = new String[questionNum];
int[] answers = new int[questionNum];
for (int i = 0; i < questionNum; i++) {
// 随机生成题目
int num1 = random.nextInt(maxNum) + 1;
int num2 = random.nextInt(maxNum) + 1;
String operator = operators[random.nextInt(operators.length)];
String question = num1 + " " + operator + " " + num2 + " = ?";
questions[i] = question;
// 计算答案
switch (operator) {
case "+":
answers[i] = num1 + num2;
break;
case "-":
answers[i] = num1 - num2;
break;
case "*":
answers[i] = num1 * num2;
break;
case "/":
answers[i] = num1 / num2;
break;
}
}
// 用户答题
int score = 0; // 分数
for (int i = 0; i < questionNum; i++) {
System.out.print((i + 1) + ". " + questions[i] + " ");
int userAnswer = sc.nextInt();
if (userAnswer == answers[i]) {
System.out.println("回答正确!");
score++;
} else {
System.out.println("回答错误!正确答案为:" + answers[i]);
}
}
// 输出测试成绩
System.out.println("您的测试成绩为:" + score + "/" + questionNum);
}
}
```
使用时,可以将以上代码复制到Eclipse中的一个Java项目中,然后运行即可。程序会要求用户输入题目数量,然后随机生成指定数量的四则运算题目,用户需要输入答案,程序会自动判断对错,并输出最终测试成绩。
阅读全文
相关推荐
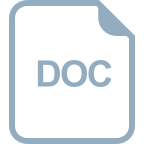
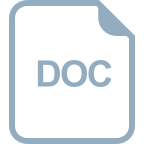
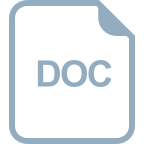
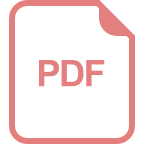
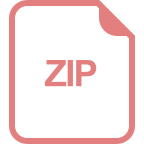
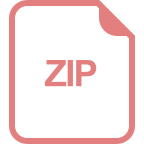
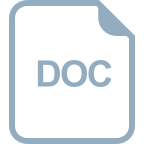
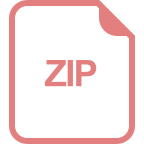
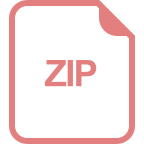
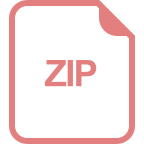
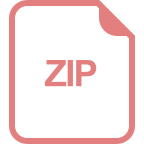
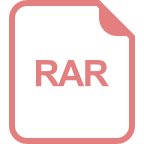
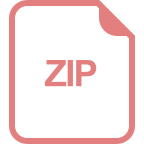
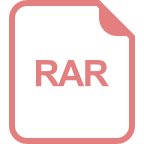
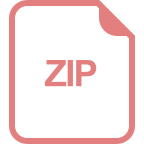
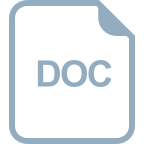