数据结构——迷宫问题用C++完整版
时间: 2023-09-02 18:15:47 浏览: 128
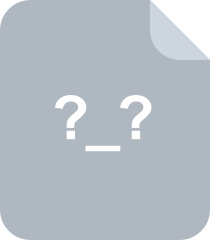
迷宫问题,C++,数据结构
以下是使用 C 语言实现迷宫问题的完整代码:
```c
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#define MAX_ROW 5
#define MAX_COL 5
int maze[MAX_ROW][MAX_COL] = {
{0, 1, 0, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 0, 0, 0},
{0, 1, 1, 1, 0},
{0, 0, 0, 1, 0},
};
void print_maze()
{
int i, j;
for (i = 0; i < MAX_ROW; i++) {
for (j = 0; j < MAX_COL; j++) {
printf("%d ", maze[i][j]);
}
printf("\n");
}
printf("*********\n");
}
typedef struct {
int row, col;
} element;
element stack[512];
int top = -1;
void push(element item)
{
stack[++top] = item;
}
element pop()
{
return stack[top--];
}
int is_empty()
{
return top == -1;
}
void path()
{
int i, row, col, next_row, next_col;
element position;
maze[0][0] = 2;
top = 0;
stack[0].row = stack[0].col = 0;
while (!is_empty()) {
position = pop();
row = position.row;
col = position.col;
printf("(%d, %d)\n", row, col);
if (row == MAX_ROW - 1 && col == MAX_COL - 1) {
printf("Path found!\n");
return;
}
if (col+1 < MAX_COL && maze[row][col+1] == 0) { // right
next_row = row;
next_col = col+1;
maze[next_row][next_col] = 2;
position.row = next_row;
position.col = next_col;
push(position);
}
if (row+1 < MAX_ROW && maze[row+1][col] == 0) { // down
next_row = row+1;
next_col = col;
maze[next_row][next_col] = 2;
position.row = next_row;
position.col = next_col;
push(position);
}
if (col-1 >= 0 && maze[row][col-1] == 0) { // left
next_row = row;
next_col = col-1;
maze[next_row][next_col] = 2;
position.row = next_row;
position.col = next_col;
push(position);
}
if (row-1 >= 0 && maze[row-1][col] == 0) { // up
next_row = row-1;
next_col = col;
maze[next_row][next_col] = 2;
position.row = next_row;
position.col = next_col;
push(position);
}
print_maze();
}
printf("Path not found\n");
}
int main()
{
path();
return 0;
}
```
在上述代码中,我们定义了一个 `maze` 数组来表示迷宫,0 表示可以通过的路,1 表示障碍物,2 表示走过的路程。我们使用栈来记录已经走过的路径,并通过 `push()` 和 `pop()` 操作来实现栈的基本功能。在 `path()` 函数中,我们从起点开始遍历迷宫,如果找到了终点,则输出 "Path found!",否则输出 "Path not found"。
我们可以通过调用 `print_maze()` 函数来输出迷宫的状态,以及调用 `path()` 函数来寻找迷宫的路径。
阅读全文
相关推荐
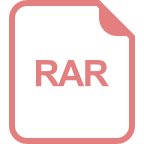
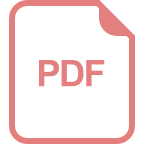
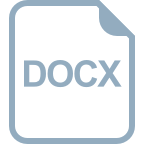
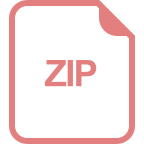
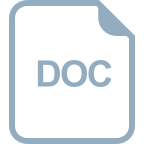
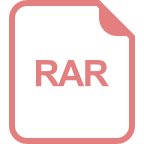
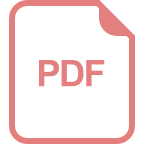
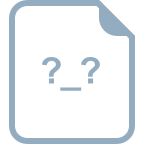
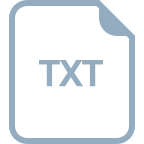
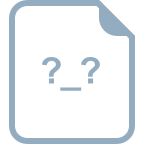
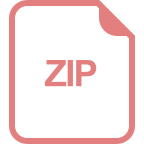