C# 用windows11 API 实现HID蓝牙键盘的链接、数据读取
时间: 2024-03-11 17:48:01 浏览: 318
好的,以下是一个简单的 C# 代码示例,用于连接 HID 蓝牙键盘并读取数据。请注意,这是一个简单的示例,仅用于演示如何使用 Windows 11 API 连接和读取 HID 蓝牙键盘数据。您需要根据您的具体情况进行修改和调整。
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Windows.Devices.Bluetooth;
using Windows.Devices.Bluetooth.GenericAttributeProfile;
namespace BluetoothKeyboard
{
class Program
{
static async Task Main(string[] args)
{
// 获取 Bluetooth 设备
var devices = await BluetoothDevice.GetPairedDevicesAsync();
// 获取 HID 蓝牙键盘的 Service UUID
var hidServiceUuid = new Guid("{00001812-0000-1000-8000-00805f9b34fb}");
// 遍历所有配对设备,并连接 HID 蓝牙键盘
foreach (var device in devices)
{
var services = await device.GetGattServicesForUuidAsync(hidServiceUuid);
if (services.Count > 0)
{
var service = services[0];
var characteristics = await service.GetCharacteristicsAsync();
// 读取 HID 蓝牙键盘数据
var dataCharacteristic = characteristics.FirstOrDefault(c => c.Uuid == new Guid("{00002a4d-0000-1000-8000-00805f9b34fb}"));
if (dataCharacteristic != null)
{
dataCharacteristic.ValueChanged += DataCharacteristic_ValueChanged;
await dataCharacteristic.WriteClientCharacteristicConfigurationDescriptorAsync(GattClientCharacteristicConfigurationDescriptorValue.Notify);
}
}
}
Console.ReadKey();
}
private static void DataCharacteristic_ValueChanged(GattCharacteristic sender, GattValueChangedEventArgs args)
{
// 处理 HID 蓝牙键盘数据
var data = args.CharacteristicValue.ToArray();
Console.WriteLine($"Received {data.Length} bytes of data.");
}
}
}
```
请注意,此代码需要在支持 Bluetooth 5.0 的 Windows 11 设备上运行。您还需要在 Visual Studio 中添加对 `Windows.Devices.Bluetooth` 和 `Windows.Devices.Bluetooth.GenericAttributeProfile` 命名空间的引用,以及在应用程序的清单文件中声明对 Bluetooth 权限的访问。
阅读全文
相关推荐
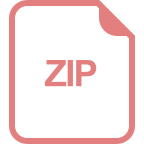
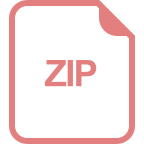















