antd hooks手动清空auoComplete组件内容
时间: 2024-03-09 11:48:19 浏览: 85
要手动清空 `AutoComplete` 组件的内容,你可以使用 `useRef` 钩子来获取 `AutoComplete` 组件的实例,然后调用其方法进行清空。
以下是示例代码:
```jsx
import React, { useRef } from 'react';
import { AutoComplete } from 'antd';
const MyComponent = () => {
const autoCompleteRef = useRef();
const handleClearAutoComplete = () => {
autoCompleteRef.current.setState({ value: '' });
};
return (
<div>
<AutoComplete ref={autoCompleteRef} />
<button onClick={handleClearAutoComplete}>清空</button>
</div>
);
};
```
在上述代码中,我们使用 `useRef` 钩子创建了一个 `autoCompleteRef` 引用,然后将其传递给 `AutoComplete` 组件的 `ref` 属性。接着,在按钮的 `onClick` 事件处理函数中,我们调用 `autoCompleteRef.current.setState` 方法来将 `AutoComplete` 组件的值清空。
相关问题
antd select 手动赋值
antd select是一个常用的下拉选择框,用于在一组选项中进行选择。当我们需要在代码中手动赋值时,可以使用select的value属性来实现。
首先,我们需要设置一个state来存储当前选中的值,例如:
```
state = {
selectedValue: null
}
```
然后,在select组件中,我们可以设置value属性,将选中的值赋给它,例如:
```
<Select value={this.state.selectedValue} onChange={this.handleChange}>
<Option value="option1">选项1</Option>
<Option value="option2">选项2</Option>
</Select>
```
其中,onChange是一个回调函数,当选中的值发生变化时,会触发这个函数。我们可以在这个函数中更新selectedValue的值,例如:
```
handleChange = (value) => {
this.setState({
selectedValue: value
})
}
```
这样,当我们需要手动赋值时,只需要更新state中的selectedValue即可,select组件会自动将其选中。例如:
```
this.setState({
selectedValue: 'option1'
})
```
这样,下拉选择框就会选中选项1。通过这种方式,我们可以在代码中方便地控制下拉选择框的选中值。
antd切换清空input输入框内容
您可以在antd的Input组件上加上一个按钮,然后在按钮的onClick事件中清空Input的值。以下是示例代码:
```
import React, { useState } from 'react';
import { Input, Button } from 'antd';
const Example = () => {
const [value, setValue] = useState('');
const clearInput = () => {
setValue('');
};
return (
<>
<Input value={value} onChange={(e) => setValue(e.target.value)} />
<Button onClick={clearInput}>清空</Button>
</>
);
};
export default Example;
```
在这个示例中,我们使用了useState hook来管理Input的值。然后在按钮的onClick事件中调用clearInput函数来清空Input的值。
阅读全文
相关推荐
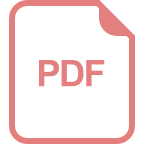
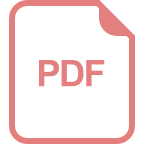
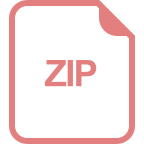
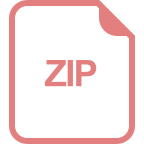
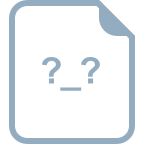
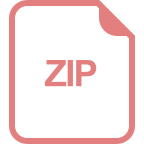
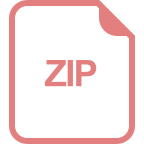
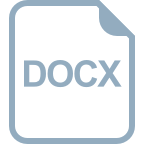








