Access 判断是否重复
时间: 2024-03-14 22:46:42 浏览: 22
在 Access 数据库中,可以使用 SQL 语句来判断是否有重复记录。具体的方法如下:
1. 打开 Access 数据库,打开要判断重复的表格。
2. 在查询设计视图中,添加要判断的字段。
3. 在查询设计视图中,选择查询→删除重复记录。
4. 在查询设计视图中,选择查询→运行,即可查看是否有重复记录。
如果需要在代码中进行判断,可以使用 OleDbDataAdapter 和 DataSet 来读取表格数据,然后遍历 DataSet 中的数据,逐个比较是否有重复数据。具体的代码如下:
```csharp
string sql = "SELECT * FROM 表格名";
OleDbDataAdapter dataAdapter = new OleDbDataAdapter(sql, 连接字符串);
DataSet dataSet = new DataSet();
dataAdapter.Fill(dataSet);
foreach (DataRow row in dataSet.Tables[0].Rows)
{
// 逐个比较是否有重复数据
}
```
在比较时,可以使用相应字段的 Equals 方法或者 CompareTo 方法来进行比较。如果发现有重复数据,可以根据具体业务需求进行相应的处理。
相关问题
vc++6.0mfc判断access添加是否重复
可以使用ADO库来判断Access中添加的记录是否重复。具体步骤如下:
1. 引入ADO库
在VC++ 6.0中,打开工程属性页,选择“Link”选项卡,然后在“Object/Library Modules”中添加“msado15.lib”。
2. 使用ADO连接Access数据库
在程序中使用ADO连接Access数据库,代码如下:
```
#include <iostream>
#include <string>
#include <comutil.h>
#import "C:\Program Files\Common Files\System\ado\msado15.dll" no_namespace rename("EOF", "adoEOF")
using namespace std;
int main()
{
_bstr_t bstrConnect = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=test.mdb";
_ConnectionPtr pConnection = NULL;
try
{
CoInitialize(NULL);
pConnection.CreateInstance("ADODB.Connection");
pConnection->Open(bstrConnect, "", "", adConnectUnspecified);
cout << "Connect to Access database successfully." << endl;
}
catch (_com_error e)
{
cout << "Failed to connect to Access database. Error message: " << e.ErrorMessage() << endl;
}
return 0;
}
```
其中,`bstrConnect`变量保存了连接字符串,指定了Access数据库的路径和提供程序。`_ConnectionPtr`是ADO库中的一个智能指针,用于管理连接对象。`CreateInstance`方法用于创建连接对象,并指定连接字符串。`Open`方法用于打开数据库连接。
3. 使用SQL语句查询记录
在程序中使用SQL语句查询Access数据库中的记录,代码如下:
```
_RecordsetPtr pRecordset = NULL;
_bstr_t bstrSQL = "SELECT * FROM table1 WHERE field1 = 'value1'";
try
{
pRecordset.CreateInstance("ADODB.Recordset");
pRecordset->Open(bstrSQL, pConnection.GetInterfacePtr(), adOpenStatic, adLockOptimistic, adCmdText);
cout << "Execute SQL statement successfully." << endl;
}
catch (_com_error e)
{
cout << "Failed to execute SQL statement. Error message: " << e.ErrorMessage() << endl;
}
if (pRecordset->adoEOF)
{
cout << "Record not found." << endl;
}
else
{
cout << "Record found." << endl;
}
pRecordset->Close();
```
其中,`_RecordsetPtr`是ADO库中的一个智能指针,用于管理记录集对象。`Open`方法用于执行SQL语句,并返回记录集对象。`adoEOF`属性指示记录集是否已到达文件结尾。如果查询到记录,则`adoEOF`为`false`,否则为`true`。
4. 判断记录是否重复
在程序中使用SQL语句查询Access数据库中的记录,如果查询到记录,则说明添加的记录已经存在,代码如下:
```
_RecordsetPtr pRecordset = NULL;
_bstr_t bstrSQL = "SELECT * FROM table1 WHERE field1 = 'value1'";
try
{
pRecordset.CreateInstance("ADODB.Recordset");
pRecordset->Open(bstrSQL, pConnection.GetInterfacePtr(), adOpenStatic, adLockOptimistic, adCmdText);
}
catch (_com_error e)
{
cout << "Failed to execute SQL statement. Error message: " << e.ErrorMessage() << endl;
}
if (pRecordset->adoEOF)
{
// add record
cout << "Record added successfully." << endl;
}
else
{
// record exists
cout << "Record already exists." << endl;
}
pRecordset->Close();
```
其中,`field1`是需要判断的字段,`value1`是需要判断的值。如果查询到了记录,则说明添加的记录已经存在。如果没有查询到记录,则可以添加新记录。
vc++6.0mfc判断access添加记录是否重复
你可以使用ADO(ActiveX Data Objects)来判断 Access 数据库中是否已经存在相同的记录。以下是示例代码:
```cpp
#include <afxdao.h>
// 假设你已经连接到了数据库并打开了相关的表
// 创建一个记录集
CDaoRecordset rs(&theDatabase);
// 设置查询语句
CString strSQL;
strSQL.Format("SELECT * FROM YourTableName WHERE YourFieldName='%s'", YourFieldValue);
// 执行查询
rs.Open(dbOpenSnapshot, strSQL);
// 判断是否存在相同的记录
if (rs.IsEOF())
{
// 不存在相同的记录,可以添加新的记录
}
else
{
// 存在相同的记录,不能添加重复的记录
}
// 关闭记录集
rs.Close();
```
在上面的代码中,`YourTableName` 表示要查询的表名,`YourFieldName` 表示要查询的字段名,`YourFieldValue` 表示要查询的字段值。你需要将它们替换为你实际的表名、字段名和字段值。如果查询结果为空,则说明不存在相同的记录,可以添加新的记录;否则说明存在相同的记录,不能添加重复的记录。
相关推荐
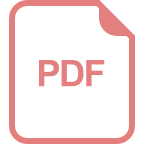
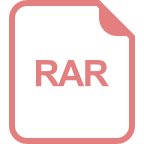












