uniapp 搜索附近位置
时间: 2023-08-16 14:03:43 浏览: 228
要实现在uniapp中搜索附近位置,可以使用高德地图的JavaScript SDK。以下是实现的步骤:
1. 在高德地图官网申请开发者账号,并创建应用获取开发者key。
2. 在uniapp项目中安装高德地图JavaScript SDK,可以使用npm安装:npm install @amap/amap-jsapi-loader --save
3. 在需要使用地图的页面中,引入高德地图JavaScript SDK并初始化地图组件:
```
<template>
<view class="map">
<map :longitude="longitude" :latitude="latitude" :markers="markers" :scale="scale" style="width: 100%; height: 100%;" />
</view>
</template>
<script>
import { createApp } from 'vue'
import { Map, Marker } from 'vue3-amap'
import AMapLoader from '@amap/amap-jsapi-loader'
export default {
components: {
Map,
Marker
},
data() {
return {
longitude: 116.397428,
latitude: 39.90923,
markers: [],
scale: 14
}
},
async mounted() {
await AMapLoader.load({
key: 'your amap key',
version: '2.0',
plugins: ['AMap.Geolocation']
})
this.initMap()
},
methods: {
async initMap() {
const geolocation = new AMap.Geolocation({
enableHighAccuracy: true, // 是否使用高精度定位,默认:true
timeout: 10000, // 超过10秒后停止定位,默认:无穷大
maximumAge: 0, // 定位结果缓存0毫秒,默认:0
convert: true, // 自动偏移坐标,偏移后的坐标为高德坐标,默认:true
showButton: true, // 显示定位按钮,默认:true
buttonPosition: 'LB', // 定位按钮停靠位置,默认:'LB',左下角
buttonOffset: new AMap.Pixel(10, 20), // 定位按钮距离容器左下角的偏移量,默认:Pixel(10, 20)
showMarker: true, // 定位成功后在定位到的位置显示点标记,默认:true
showCircle: true, // 定位成功后用圆圈表示定位精度范围,默认:true
panToLocation: true, // 定位成功后将定位到的位置作为地图中心点,默认:true
zoomToAccuracy: true // 定位成功后调整地图视野范围使定位位置及精度范围完全显示,默认:false
})
const map = new AMap.Map('map', {
zoom: 14,
center: [116.397428, 39.90923],
resizeEnable: true
})
map.addControl(geolocation)
map.plugin('AMap.Geolocation', function() {
geolocation.getCurrentPosition()
AMap.event.addListener(geolocation, 'complete', onComplete)
AMap.event.addListener(geolocation, 'error', onError)
})
const that = this
function onComplete(data) {
that.longitude = data.position.lng
that.latitude = data.position.lat
that.searchNearby(data.position)
}
function onError(data) {
console.log(data)
}
},
async searchNearby(lnglat) {
const that = this
AMap.plugin('AMap.PlaceSearch', function() {
const placeSearch = new AMap.PlaceSearch({
pageSize: 10,
pageIndex: 1,
city: '全国',
map: that.map,
panel: 'panel'
})
placeSearch.searchNearBy('', lnglat, 1000, function(status, result) {
if (status === 'complete') {
const pois = result.poiList.pois
const markers = pois.map(poi => {
return {
position: [poi.location.lng, poi.location.lat],
title: poi.name,
iconPath: '../../static/images/marker.png'
}
})
that.markers = markers
}
})
})
}
}
}
</script>
<style>
.map {
width: 100%;
height: 100%;
}
</style>
```
在以上代码中,首先使用AMapLoader加载高德地图JavaScript SDK,并在mounted生命周期中初始化地图组件。在initMap方法中,使用AMap.Geolocation获取当前位置,然后调用searchNearby方法搜索附近位置。在searchNearby方法中,使用AMap.PlaceSearch搜索附近位置,并将搜索结果标注在地图上。
以上是实现在uniapp中搜索附近位置的基本步骤,可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
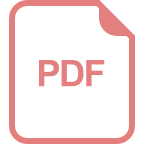


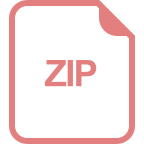
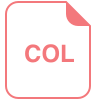


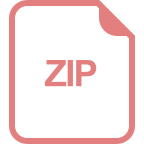
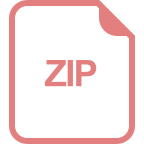
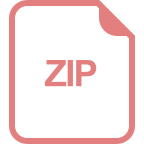
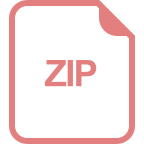
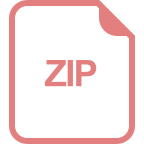
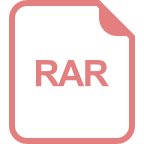
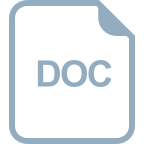
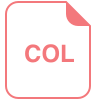
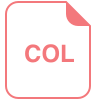
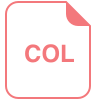