java 程序阻塞时如何让他等待几秒后抛出异常
时间: 2024-05-07 10:18:15 浏览: 13
你可以使用 Java 中的 `Thread.sleep()` 方法来让程序等待一定的时间后抛出异常。具体实现可以参考以下代码:
```java
try {
// 要执行的代码
} catch (Exception e) {
try {
Thread.sleep(5000); // 等待 5 秒
throw new Exception("执行超时"); // 抛出异常
} catch (InterruptedException ex) {
ex.printStackTrace();
}
}
```
在 `try` 代码块中,如果程序阻塞了,就会跳到 `catch` 代码块中。在 `catch` 中,先使用 `Thread.sleep()` 方法让程序等待 5 秒,然后再抛出一个异常。这样就可以实现在程序阻塞时等待一段时间后抛出异常的效果。
相关问题
Java实现30s后结束程序
### 回答1:
可以使用Thread.sleep()方法来让Java程序暂停一段时间,结合System.exit()方法来结束程序。以下是一个示例代码:
```
public class Main {
public static void main(String[] args) {
try {
Thread.sleep(30000); // 暂停30s
} catch (InterruptedException e) {
e.printStackTrace();
}
System.exit(0); // 结束程序
}
}
```
在30秒后,程序将自动退出。
### 回答2:
要实现Java程序在30秒后结束,可以使用Thread类的sleep方法来使程序暂停执行。我们可以创建一个单独的线程,在执行完指定的时间后调用System.exit(0)方法来结束程序。
下面是实现的示例代码:
```java
public class Main {
public static void main(String[] args) {
Thread thread = new Thread(() -> {
try {
Thread.sleep(30000); // 暂停执行30秒
System.exit(0); // 结束程序
} catch (InterruptedException e) {
e.printStackTrace();
}
});
thread.start();
// 在这里可以写下你的其他代码,这些代码将会在30秒后程序结束之前执行
// 例如:
System.out.println("程序开始执行");
for (int i = 0; i < 10; i++) {
System.out.println("执行第" + (i + 1) + "次");
try {
Thread.sleep(1000); // 暂停1秒
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// 等待30秒结束线程
try {
thread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("程序结束");
}
}
```
在上述代码中,我们创建了一个新的线程,在该线程中使用Thread.sleep方法使其暂停执行30秒,然后调用System.exit(0)方法结束程序。在主线程中,我们执行了一些其他代码,这些代码将在30秒后程序结束之前执行。最后,我们使用thread.join()方法等待30秒,确保线程执行完毕后程序才结束。
### 回答3:
在Java中,可以使用`Thread.sleep()`方法来实现程序在指定时间后自动结束。
下面是一个示例代码段,展示了如何在30秒后结束程序:
```java
public class Main {
public static void main(String[] args) {
try {
// 暂停程序执行 30 秒
Thread.sleep(30000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// 30 秒后的代码执行
System.out.println("程序已结束");
}
}
```
在上面的代码中,我们在`main()`方法中使用了`Thread.sleep(30000)`来暂停程序执行30秒。当30秒过去后,程序将恢复执行,执行下一行代码,将"程序已结束"打印到控制台。
需要注意的是,`Thread.sleep()`方法会抛出`InterruptedException`异常,所以我们需要进行异常处理或者简单地使用`e.printStackTrace()`输出异常信息。
另外,为了避免程序在运行期间阻塞,在进行长时间的等待之前,我们可以将这段代码放在一个线程中。这样可以避免阻塞主线程,例如:
```java
public class Main {
public static void main(String[] args) {
Thread thread = new Thread(() -> {
try {
// 暂停程序执行 30 秒
Thread.sleep(30000);
} catch (InterruptedException e) {
e.printStackTrace();
}
// 30 秒后的代码执行
System.out.println("程序已结束");
});
thread.start();
}
}
```
在这个示例中,我们将等待的逻辑放入一个新的线程,并使用`thread.start()`方法启动线程执行。这样可以避免主线程的阻塞,并允许其他的代码在等待的同时继续执行。
Java中的ExecutorService executor 等待所有线程结束
在Java中,ExecutorService提供了awaitTermination()方法来等待所有线程执行完成。该方法会阻塞当前线程,直到所有线程执行完成或者超时。下面是一个示例代码:
```java
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
public class WaitAllThreadsDemo {
private static ExecutorService executor = Executors.newFixedThreadPool(2);
public static void main(String[] args) throws InterruptedException {
executor.submit(new Runnable() {
@Override
public void run() {
System.out.println("Thread 1 started");
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread 1 ended");
}
});
executor.submit(new Runnable() {
@Override
public void run() {
System.out.println("Thread 2 started");
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Thread 2 ended");
}
});
executor.shutdown();
executor.awaitTermination(10, TimeUnit.SECONDS);
System.out.println("All threads ended");
}
}
```
在上面的示例代码中,我们创建了一个ExecutorService对象,并使用submit()方法提交了两个Runnable任务。然后,我们调用ExecutorService.shutdown()方法来关闭线程池,并使用ExecutorService.awaitTermination()方法来等待所有线程执行完成。我们设置了10秒的超时时间,如果超过该时间仍有线程没有执行完成,则会抛出InterruptedException异常。
需要注意的是,在使用ExecutorService等待所有线程执行完成时,要注意线程之间的同步问题,以避免出现线程安全问题。同时,也要注意设置合适的超时时间,以避免等待时间过长导致程序阻塞。
相关推荐
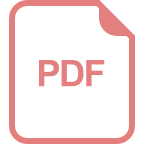
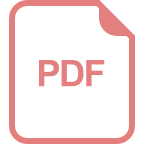
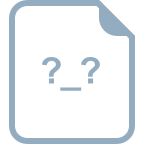












