自己定义一个异常类,继承Exception类, 捕获下面的过程:判断输入的字符串长度是否小于5,如果小于5,比如输入长度为3则输出:" The input is of length 3,expecting at least 5',大于5输出"print success'。
时间: 2023-11-27 07:53:53 浏览: 27
可以定义如下的异常类,并按照要求进行捕获和处理:
```python
class InputLengthError(Exception):
def __init__(self, length):
self.length = length
def __str__(self):
return f"The input is of length {self.length}, expecting at least 5"
```
然后在程序中使用 try-except 捕获该异常,并根据异常对象的内容做出相应的处理:
```python
try:
s = input("Please enter a string: ")
if len(s) < 5:
raise InputLengthError(len(s))
else:
print("Success!")
except InputLengthError as e:
print(e)
```
这样,当输入字符串的长度小于5时,会触发 `InputLengthError` 异常,程序会输出对应的提示信息;当输入字符串的长度大于等于5时,会正常输出“Success!”。
相关问题
phython自己定义一个异常类,继承Exception类, 捕获下面的过程:判断输入的字符串长度是否小于5,如果小于5,比如输入长度为3则输出:" The input is of length 3,expecting at least 5',大于5输出"print success'。
可以使用以下代码实现:
```python
class InputLengthError(Exception):
pass
def check_input_length(input_str):
if len(input_str) < 5:
raise InputLengthError(f"The input is of length {len(input_str)}, expecting at least 5")
else:
print("print success")
try:
input_str = input("Please input a string: ")
check_input_length(input_str)
except InputLengthError as e:
print(e)
```
首先定义了一个继承自Exception的异常类InputLengthError,用于在输入长度小于5时抛出异常。
然后定义了一个check_input_length函数,用于检查输入字符串的长度。如果长度小于5,则抛出InputLengthError异常,否则输出"print success"。
最后,在try语句块中调用check_input_length函数,并在except语句块中捕获InputLengthError异常并输出错误信息。
自定义一个异常类继承Exception类,捕获下面的过程:判断输入的字符串长度是否小于5,如果小于5,比如长度为3,则输出“The input is of length 3,expecting at least 5!”,如果大于5,则输出“Print success!”。
可以参考下面的代码实现:
```python
class InputLengthError(Exception):
def __init__(self, length):
self.length = length
def __str__(self):
return f"The input is of length {self.length}, expecting at least 5!"
try:
s = input("Please enter a string: ")
if len(s) < 5:
raise InputLengthError(len(s))
else:
print("Print success!")
except InputLengthError as e:
print(e)
```
首先我们自定义了一个异常类`InputLengthError`,它继承了`Exception`类。在类的初始化函数中,我们保存了输入字符串的长度。在`__str__`函数中,我们定义了当这个异常被打印时所输出的字符串。
在程序的主体部分,我们首先通过`input`函数获取了用户输入的字符串`s`。然后我们使用`if`语句判断`s`的长度是否小于5。如果小于5,我们手动抛出了一个`InputLengthError`异常,并传入了`s`的长度作为参数。如果`s`的长度大于等于5,我们就直接输出了“Print success!”。在`try-except`语句中,我们通过`except`捕获了`InputLengthError`异常,并打印了异常对象的字符串表示。
阅读全文
相关推荐
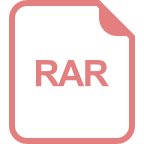





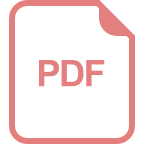
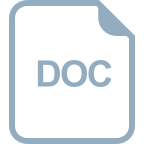
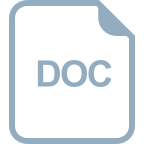
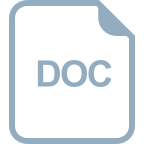
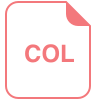
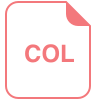
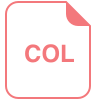
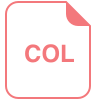