数据库电影院售票管理系统课程设计代码
时间: 2024-12-10 18:14:10 浏览: 18
以下是一个简单的数据库电影院售票管理系统的课程设计代码示例,使用Python和SQLite数据库实现:
```python
import sqlite3
# 连接到SQLite数据库(如果数据库不存在,则会自动创建)
conn = sqlite3.connect('cinema.db')
cursor = conn.cursor()
# 创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS movies (
id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT NOT NULL,
duration INTEGER NOT NULL,
description TEXT
)
''')
cursor.execute('''
CREATE TABLE IF NOT EXISTS showtimes (
id INTEGER PRIMARY KEY AUTOINCREMENT,
movie_id INTEGER NOT NULL,
time TEXT NOT NULL,
FOREIGN KEY (movie_id) REFERENCES movies(id)
)
''')
cursor.execute('''
CREATE TABLE IF NOT EXISTS tickets (
id INTEGER PRIMARY KEY AUTOINCREMENT,
showtime_id INTEGER NOT NULL,
seat TEXT NOT NULL,
price REAL NOT NULL,
FOREIGN KEY (showtime_id) REFERENCES showtimes(id)
)
''')
# 插入一些示例数据
movies = [
('The Shawshank Redemption', 142, 'Two imprisoned men bond over a number of years, finding solace and eventual redemption through acts of common decency.'),
('The Godfather', 175, 'The aging patriarch of an organized crime dynasty transfers control of his clandestine empire to his reluctant son.'),
('The Dark Knight', 152, 'When the menace known as the Joker wreaks havoc and chaos on the people of Gotham, Batman must accept one of the greatest psychological and physical tests of his ability to fight injustice.')
]
cursor.executemany('INSERT INTO movies (title, duration, description) VALUES (?, ?, ?)', movies)
showtimes = [
(1, '19:00'),
(1, '21:30'),
(2, '18:30'),
(2, '22:00'),
(3, '20:00'),
(3, '23:30')
]
cursor.executemany('INSERT INTO showtimes (movie_id, time) VALUES (?, ?)', showtimes)
conn.commit()
# 查询所有电影
def get_all_movies():
cursor.execute('SELECT * FROM movies')
return cursor.fetchall()
# 查询所有场次
def get_all_showtimes():
cursor.execute('SELECT s.id, m.title, s.time FROM showtimes s JOIN movies m ON s.movie_id = m.id')
return cursor.fetchall()
# 查询某个场次的座位
def get_seats(showtime_id):
cursor.execute('SELECT seat FROM tickets WHERE showtime_id = ?', (showtime_id,))
return [row[0] for row in cursor.fetchall()]
# 购买电影票
def buy_ticket(showtime_id, seat, price):
cursor.execute('INSERT INTO tickets (showtime_id, seat, price) VALUES (?, ?, ?)', (showtime_id, seat, price))
conn.commit()
# 关闭数据库连接
def close_connection():
conn.close()
# 示例使用
if __name__ == "__main__":
print("所有电影:")
for movie in get_all_movies():
print(movie)
print("\n所有场次:")
for showtime in get_all_showtimes():
print(showtime)
# 假设我们要购买第一场次的第5排3座
showtime_id = 1
seat = "5-3"
price = 50.0
if seat not in get_seats(showtime_id):
buy_ticket(showtime_id, seat, price)
print(f"\n购买成功!你已购买{showtime_id}号场次的{seat}座,价格为{price}元。")
else:
print("\n抱歉,这个座位已经被预订了。")
close_connection()
```
这个示例展示了如何创建一个基本的电影院售票管理系统,包括以下功能:
1. 创建数据库和表结构
2. 插入示例数据
3. 查询所有电影
4. 查询所有场次
5. 查询某个场次的座位
6. 购买电影票
阅读全文
相关推荐
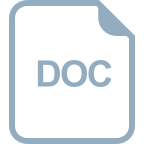
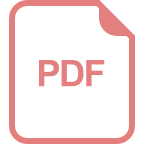

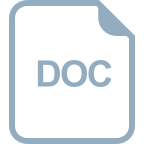

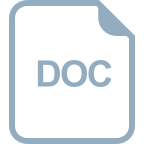
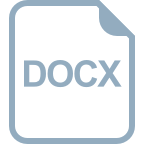
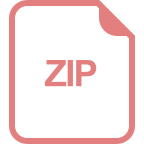
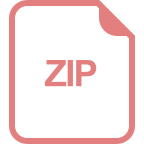
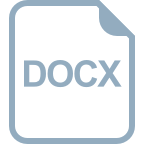
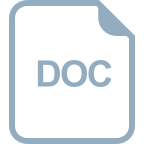
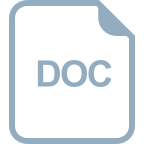
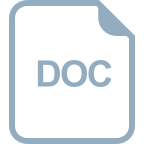
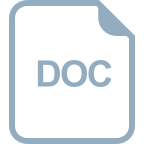
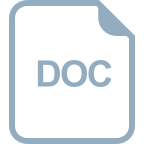

