用Android实现商品详情页。 要求: 1. 点击某一个商品进入本商品的详情页,详情页数据包括商品图片、商品名称、商品价格、该商品的详细介绍。 2. 商品详情页底部包含一个“购买”按钮,点击购买后,跳转到购物车页面。 3. 购物车页面可实现商品数量的增减、商品的删除以及总价格计算功能。给出详细代码,adapter要在新建类中
时间: 2024-02-16 16:03:24 浏览: 114
好的,以下是一个简单的实现方案,供参考:
1. 商品详情页布局文件(item_product_detail.xml):
```
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/iv_product_image"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@drawable/product_image"/>
<TextView
android:id="@+id/tv_product_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/iv_product_image"
android:text="商品名称"/>
<TextView
android:id="@+id/tv_product_price"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/tv_product_name"
android:text="商品价格"/>
<TextView
android:id="@+id/tv_product_desc"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/tv_product_price"
android:text="商品介绍"/>
<Button
android:id="@+id/btn_buy"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:text="购买"/>
</RelativeLayout>
```
2. 商品详情页适配器(ProductDetailAdapter.java):
```
public class ProductDetailAdapter extends RecyclerView.Adapter<ProductDetailAdapter.ViewHolder> {
private Context mContext;
public ProductDetailAdapter(Context context) {
mContext = context;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(mContext).inflate(R.layout.item_product_detail, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
// 绑定数据
holder.ivProductImage.setImageResource(R.drawable.product_image);
holder.tvProductName.setText("商品名称");
holder.tvProductPrice.setText("商品价格");
holder.tvProductDesc.setText("商品介绍");
// 设置购买按钮点击事件
holder.btnBuy.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// 跳转到购物车页面
Intent intent = new Intent(mContext, ShoppingCartActivity.class);
mContext.startActivity(intent);
}
});
}
@Override
public int getItemCount() {
return 1;
}
static class ViewHolder extends RecyclerView.ViewHolder {
ImageView ivProductImage;
TextView tvProductName;
TextView tvProductPrice;
TextView tvProductDesc;
Button btnBuy;
ViewHolder(@NonNull View itemView) {
super(itemView);
ivProductImage = itemView.findViewById(R.id.iv_product_image);
tvProductName = itemView.findViewById(R.id.tv_product_name);
tvProductPrice = itemView.findViewById(R.id.tv_product_price);
tvProductDesc = itemView.findViewById(R.id.tv_product_desc);
btnBuy = itemView.findViewById(R.id.btn_buy);
}
}
}
```
3. 购物车页面布局文件(activity_shopping_cart.xml):
```
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/tv_total_price"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="总价:0"/>
<RecyclerView
android:id="@+id/rv_product_list"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@id/tv_total_price"/>
</RelativeLayout>
```
4. 购物车页面适配器(ShoppingCartAdapter.java):
```
public class ShoppingCartAdapter extends RecyclerView.Adapter<ShoppingCartAdapter.ViewHolder> {
private List<Product> mProductList = new ArrayList<>();
public void setData(List<Product> productList) {
mProductList.clear();
mProductList.addAll(productList);
notifyDataSetChanged();
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_shopping_cart, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
Product product = mProductList.get(position);
holder.tvProductName.setText(product.getName());
holder.tvProductPrice.setText(String.valueOf(product.getPrice()));
holder.tvProductCount.setText(String.valueOf(product.getCount()));
holder.btnDelete.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
mProductList.remove(position);
notifyDataSetChanged();
}
});
}
@Override
public int getItemCount() {
return mProductList.size();
}
static class ViewHolder extends RecyclerView.ViewHolder {
TextView tvProductName;
TextView tvProductPrice;
TextView tvProductCount;
Button btnDelete;
ViewHolder(@NonNull View itemView) {
super(itemView);
tvProductName = itemView.findViewById(R.id.tv_product_name);
tvProductPrice = itemView.findViewById(R.id.tv_product_price);
tvProductCount = itemView.findViewById(R.id.tv_product_count);
btnDelete = itemView.findViewById(R.id.btn_delete);
}
}
}
```
5. 购物车页面实现(ShoppingCartActivity.java):
```
public class ShoppingCartActivity extends AppCompatActivity {
private RecyclerView mRecyclerView;
private ShoppingCartAdapter mAdapter;
private TextView mTvTotalPrice;
private List<Product> mProductList = new ArrayList<>();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_shopping_cart);
mTvTotalPrice = findViewById(R.id.tv_total_price);
mRecyclerView = findViewById(R.id.rv_product_list);
mRecyclerView.setLayoutManager(new LinearLayoutManager(this));
mAdapter = new ShoppingCartAdapter();
mRecyclerView.setAdapter(mAdapter);
initData();
}
private void initData() {
mProductList.add(new Product("商品1", 10.0, 1));
mProductList.add(new Product("商品2", 20.0, 2));
mProductList.add(new Product("商品3", 30.0, 3));
mAdapter.setData(mProductList);
// 计算总价
double totalPrice = 0;
for (Product product : mProductList) {
totalPrice += product.getPrice() * product.getCount();
}
mTvTotalPrice.setText("总价:" + totalPrice);
}
}
```
6. 商品类(Product.java):
```
public class Product {
private String mName;
private double mPrice;
private int mCount;
public Product(String name, double price, int count) {
mName = name;
mPrice = price;
mCount = count;
}
public String getName() {
return mName;
}
public double getPrice() {
return mPrice;
}
public int getCount() {
return mCount;
}
}
```
以上是一个简单的实现方案,仅供参考。实际开发中,还需要根据实际需求进行适当的调整和优化。
阅读全文
相关推荐
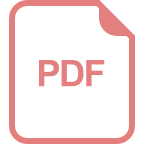
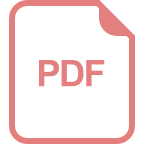










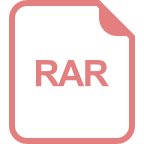
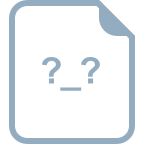
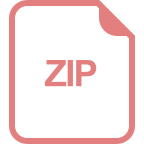