7-7 身份证读取出生地区python
时间: 2024-10-10 22:09:46 浏览: 99
在Python中,身份证号码(中国居民身份证号通常有18位)的前6位可以用来提取出生地的信息。第7-14位代表的是出生年月日。如果想通过编程的方式获取出生地区,你可以使用正则表达式来解析。
首先,你需要导入`re`模块来处理正则表达式。下面是一个简单的示例:
```python
import re
def read_birth_region(id_number):
# 定义身份证号码的正则模式
pattern = r'^(\d{6})(\d{4})(\d{2})(\d{2})$'
# 使用正则匹配并分组
match = re.match(pattern, id_number)
if match:
area_code = match.group(1) # 前六位代表省份和城市
return f"出生地区:{area_code}"
else:
return "无效的身份证号码"
# 示例身份证号码
id = '123456199001012345'
print(read_birth_region(id))
```
相关问题
使用Python读取身份证信息写入到Excel,请用Python代码实现
好的,以下是一个简短的 Python 代码示例,可用于读取身份证信息并将其写入 Excel 文件:
```python
import openpyxl
import requests
import re
import time
# 请求身份证信息接口
id_card_number = '身份证号码'
url = f'https://api.oioweb.cn/idcard/?id={id_card_number}'
response = requests.get(url)
json_data = response.json()
# 从接口返回的 JSON 数据中提取身份证信息
address = json_data['data']['address']
gender = json_data['data']['sex']
birthday = json_data['data']['birth']
# 将身份证信息写入 Excel 文件
wb = openpyxl.Workbook()
ws = wb.active
ws['B1'] = '地址'
ws['C1'] = '性别'
ws['D1'] = '出生日期'
ws['A2'] = id_card_number
ws['B2'] = address
ws['C2'] = gender
ws['D2'] = birthday
filename = 'id_cards.xlsx'
wb.save(filename)
print(f'身份证信息已经保存到 {filename} 文件中。')
```
这段代码使用了 requests 库来请求身份证信息接口,并使用 openpyxl 库将信息写入 Excel 文件。在实际使用中,需要将 `id_card_number` 变量替换为实际的身份证号码,以及确保身份证信息接口可用。
python读取身份证的信息,换一种方法
如果您想使用另一种方法来读取身份证信息,您可以考虑使用第三方的身份证识别API。这些API通常提供了更精确和稳定的身份证识别功能。
以下是使用百度AI开放平台的身份证识别API的示例代码:
首先,确保您已经在百度AI开放平台上注册并创建了一个应用,然后获取到了您的API Key和Secret Key。
然后,使用以下代码来调用百度AI的身份证识别API:
```python
import requests
# 百度AI的API接口地址
url = 'https://aip.baidubce.com/rest/2.0/ocr/v1/idcard'
# 您的API Key和Secret Key
api_key = 'Your API Key'
secret_key = 'Your Secret Key'
# 身份证图像路径
image_path = 'id_card.jpg'
# 读取身份证图像
with open(image_path, 'rb') as f:
image = f.read()
# 发送POST请求并获取识别结果
response = requests.post(url, params={'access_token': access_token}, headers={'Content-Type': 'application/x-www-form-urlencoded'}, data={'image': image, 'id_card_side': 'front'})
# 解析识别结果
result = response.json()
if 'error_code' in result:
print('身份证识别失败:', result['error_msg'])
else:
# 输出识别结果
print('姓名:', result['words_result']['姓名']['words'])
print('性别:', result['words_result']['性别']['words'])
print('民族:', result['words_result']['民族']['words'])
print('出生日期:', result['words_result']['出生']['words'])
print('住址:', result['words_result']['住址']['words'])
print('身份证号码:', result['words_result']['公民身份号码']['words'])
```
在这个示例中,我们使用了百度AI开放平台的身份证识别API。首先,我们需要在代码中填入您的API Key和Secret Key。然后,将身份证图像的路径设置为`image_path`。
接下来,我们读取身份证图像,并将其作为POST请求的参数发送到百度AI的API接口。然后,我们解析返回的识别结果,并输出身份证的各项信息。
请注意,这个示例中使用的是身份证正面识别,如果您需要识别身份证背面或其他类型的证件,请参考百度AI开放平台的文档进行相应的调整。
希望对您有所帮助!如有任何疑问,请随时提问。
阅读全文
相关推荐
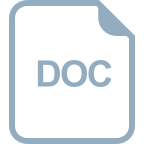
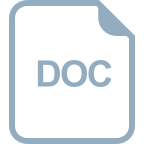
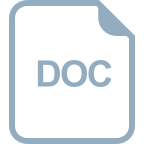
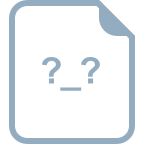
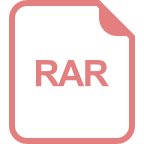
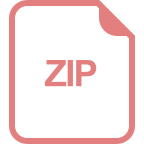
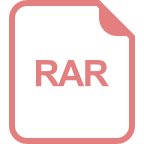
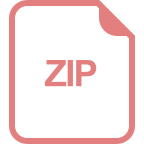
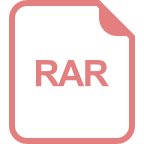
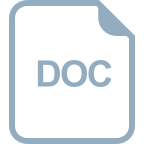
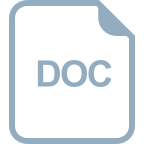
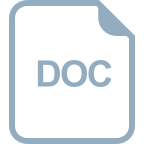
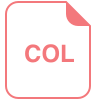



