template: ` <form @submit.prevent="login"> <div v-if="error" class="alert alert-danger">{{ error }}</div> <div class="form-group"> <label for="email">Email address</label> <input v-model="email" type="email"
时间: 2023-06-26 21:03:47 浏览: 44
该代码片段是一个Vue.js组件模板,用于显示一个登录表单。其中包含一个表单标签(通过`@submit.prevent`绑定了一个名为`login`的方法)和一些表单元素:
- 一个用于显示错误消息的div元素,通过`v-if`绑定到`error`变量,只有当`error`存在时才会显示。
- 一个表单组元素,包含一个label和一个input元素,用于输入电子邮件地址。input元素通过`v-model`绑定到组件实例中的`email`变量。
该组件的样式使用了Bootstrap框架中的alert和form-group类。
相关问题
template: ` <form @submit.prevent="login"> <div v-if="error" class="alert alert-danger">{{ error }}</div> <div class="form-group"> <label for="email">Email address</label> 如何配置这个组件
首先,你需要安装 Vue.js 。然后,你可以在你的 Vue.js 应用程序中创建一个新的组件,并将该模板复制到该组件中。在该组件的逻辑部分,你需要实现一个 `login` 方法,该方法将在提交表单时被调用。你还需要定义一个 `error` 变量,它将在登录时显示错误消息。最后,你需要在你的应用程序中使用该组件。以下是一个简单的例子:
1. 安装 Vue.js
```
npm install vue
```
2. 创建一个新的组件
```
<template>
<form @submit.prevent="login">
<div v-if="error" class="alert alert-danger">{{ error }}</div>
<div class="form-group">
<label for="email">Email address</label>
<input type="email" class="form-control" id="email" v-model="email">
</div>
<div class="form-group">
<label for="password">Password</label>
<input type="password" class="form-control" id="password" v-model="password">
</div>
<button type="submit" class="btn btn-primary">Login</button>
</form>
</template>
<script>
export default {
data() {
return {
email: '',
password: '',
error: null
}
},
methods: {
login() {
// Add your login logic here
}
}
}
</script>
```
3. 在你的应用程序中使用该组件
```
<template>
<div>
<login-form></login-form>
</div>
</template>
<script>
import LoginForm from './LoginForm.vue'
export default {
components: {
'login-form': LoginForm
}
}
</script>
```
<template> <view> <form @submit.prevent="submitForm"> <view> <label>类型:</label> <select v-model="formData.type"> <option value="income">收入</option> <option value="outcome">支出</option> </select> </view> <view> <label>金额:</label> <input type="number" v-model="formData.amount" /> </view> <view> <label>日期:</label> <input type="date" v-model="formData.date" /> </view> <button type="submit">记一笔</button> </form> </view> </template> <script setup> import { ref } from 'vue'; const formData = ref({ type: '', amount: '', date: new Date() }); function submitForm() { // 提交表单 console.log(formData.value); } </script>将代码完善,添加样式
<>
<div class="form-wrapper">
<form @submit.prevent="submitForm">
<div class="form-item">
<label>类型:</label>
<select v-model="formData.type">
<option value="income">收入</option>
<option value="outcome">支出</option>
</select>
</div>
<div class="form-item">
<label>金额:</label>
<input type="number" v-model="formData.amount" />
</div>
<div class="form-item">
<label>日期:</label>
<input type="date" v-model="formData.date" />
</div>
<button type="submit">记一笔</button>
</form>
</div>
</template>
<style scoped>
.form-wrapper {
max-width: 400px;
margin: 0 auto;
}
.form-item {
margin-bottom: 20px;
}
label {
display: inline-block;
width: 60px;
text-align: right;
margin-right: 10px;
}
</style>
<script setup>
import { ref } from 'vue';
const formData = ref({
type: '',
amount: '',
date: new Date(),
});
function submitForm() {
// 提交表单
console.log(formData.value);
}
</script>
在上面的代码中,我添加了一个 "form-wrapper" 的 div 包裹整个表单,用于设置表单最大宽度和居中对齐。我还添加了一个 "form-item" 的 div 包裹每个表单项,用于设置表单项之间的间距。最后,我添加了一些基本的样式用于对齐和布局。
相关推荐
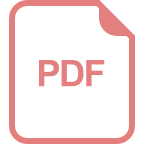
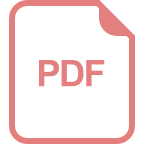

<template> 学生签到系统 <input type="text" v-model="student.S_id" placeholder="请输入学号"> <input type="text" v-model="student.S_pwd" placeholder="请输入密码"> 登录 班级尚未开启签到 <button @click="close">取消</button> </template> <script> import qs from "qs" import axios from "axios"; export default { name: "Login", data(){ return{ //弹窗 show:false, student:{ S_id:null, S_pwd:null, } } }, methods: { close() { this.show = false; // 隐藏弹出窗口 }, Login() { let param = qs.stringify(this.student); axios.post('/xx/students/login', param).then((response) => { //获取当前学生的班级信息并传入sessionStorage,参数为S_class sessionStorage.setItem('classInfo', response.data.data.s_class); //获取当前学生的id信息并传入sessionStorage sessionStorage.setItem('studentId', this.student.S_id); //弹窗 if (response.data.msg === "账号或密码错误"){ this.show = true; } if (response.data.code == 200) { this.$router.push({"path": "/signLog"}) } }).catch((error) => { console.log(error); }); } } } </script> <style scoped> .wrapper > h2{ text-align: center; margin: 40px 0px; } .content{ padding: 8px; } .form-item { margin-bottom: 20px; } .form-item > input{ width: 100%; height: 40px; padding: 4px; outline: none; border: 1px solid rebeccapurple; box-sizing: border-box; } .form-item > a{ display: inline-block; width: 100%; height: 40px; background-color: sandybrown; text-align: center; line-height: 40px; } .form-item > 这段代码要怎么样实现弹窗




图书管理 <label for="id"> 编号: </label> <input type="text" id="id" v-model='id' :disabled="flag"> <label for="name"> 名称: </label> <input type="text" id="name" v-model='name'> <button @click='handle'>提交</button> 编号 名称 时间 操作 {{item.id}} {{item.name}} {{item.date}} 修改 | 删除 用vue实现界面想要的图书信息修改,点击修改按钮后将编号传入编号输入栏并禁用,在图书名称输入栏进行名字的修改









