矩阵代码
时间: 2023-07-09 16:25:07 浏览: 253
以下是一个简单的 C++ 代码示例,用于创建和操作矩阵:
```c++
#include <iostream>
#include <vector>
using namespace std;
// 定义矩阵类
class Matrix {
private:
int rows, cols;
vector<vector<double>> data;
public:
// 构造函数
Matrix(int rows, int cols) {
this->rows = rows;
this->cols = cols;
data = vector<vector<double>>(rows, vector<double>(cols));
}
// 矩阵加法
Matrix operator+(const Matrix& other) const {
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
// 矩阵乘法
Matrix operator*(const Matrix& other) const {
Matrix result(rows, other.cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < other.cols; j++) {
double sum = 0;
for (int k = 0; k < cols; k++) {
sum += data[i][k] * other.data[k][j];
}
result.data[i][j] = sum;
}
}
return result;
}
// 打印矩阵
void print() const {
cout << "[";
for (int i = 0; i < rows; i++) {
if (i > 0) {
cout << " ";
}
cout << "[";
for (int j = 0; j < cols; j++) {
if (j > 0) {
cout << " ";
}
cout << data[i][j];
}
cout << "]";
if (i < rows - 1) {
cout << endl;
}
}
cout << "]" << endl;
}
};
int main() {
// 创建两个矩阵
Matrix A(2, 3);
A.data = {{1, 2, 3}, {4, 5, 6}};
Matrix B(3, 4);
B.data = {{7, 8, 9, 10}, {11, 12, 13, 14}, {15, 16, 17, 18}};
// 打印矩阵
A.print();
cout << endl;
B.print();
cout << endl;
// 计算矩阵乘法
Matrix C = A * B;
C.print();
cout << endl;
// 计算矩阵加法
Matrix D = A + B;
D.print();
return 0;
}
```
这段代码定义了一个 `Matrix` 类,可以创建和操作矩阵。它支持矩阵加法和乘法,并提供了一个打印矩阵的函数。在 `main` 函数中,我们创建了两个矩阵 `A` 和 `B`,并计算了它们的乘积和总和。
阅读全文
相关推荐





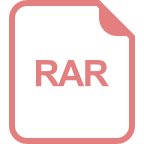
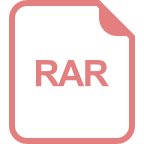
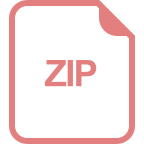



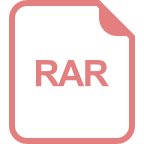