c语言中编写有参函数,实现算数运算功能,例如输入2个整数及算数运算符+ - * / %,通过调用该函数可获得算数运算结果
时间: 2023-12-14 17:39:05 浏览: 54
以下是一个示例程序,实现了输入两个整数和算数运算符,然后调用有参函数进行相应的计算,并输出结果:
```c
#include <stdio.h>
int arithmetic(int a, int b, char op)
{
switch(op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
if (b == 0) {
printf("Error: divided by zero!\n");
return 0;
}
return a / b;
case '%':
if (b == 0) {
printf("Error: divided by zero!\n");
return 0;
}
return a % b;
default:
printf("Error: invalid operator!\n");
return 0;
}
}
int main()
{
int a, b, result;
char op;
printf("Please enter two integers and an arithmetic operator (+ - * / %%):\n");
scanf("%d %d %c", &a, &b, &op);
result = arithmetic(a, b, op);
printf("The result is %d.\n", result);
return 0;
}
```
在这个程序中,`arithmetic` 函数接受三个参数,分别是两个整数和一个算数运算符。在函数内部,使用 `switch` 语句根据运算符进行相应的计算,并返回计算结果。
在 `main` 函数中,首先提示用户输入两个整数和一个算数运算符,然后调用 `arithmetic` 函数进行计算,并将结果输出。注意,在读取运算符时,需要使用 `%c` 格式说明符,因为字符无法直接使用 `%d` 或 `%f` 等格式说明符读取。
相关推荐
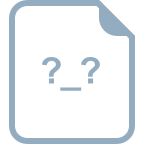
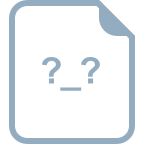
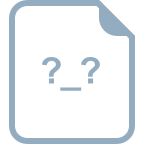














