java 怎么根据InputStream获取视频文件的类型
时间: 2023-10-25 11:14:18 浏览: 95
获取视频文件类型的一种方法是通过文件的魔数(Magic Number)来判断。魔数是文件开头的几个字节,用于标识文件类型。常见的视频文件类型和对应的魔数如下:
| 文件类型 | 魔数 |
| -------- | --- |
| AVI | 52 49 46 46 |
| MP4 | 00 00 00 18 66 74 79 70 |
| MKV | 1A 45 DF A3 |
| WMV | 30 26 B2 75 8E 66 CF 11 |
在Java中,可以通过InputStream读取文件开头的几个字节,然后与上述魔数进行比较,来判断文件类型。示例代码如下:
```java
import java.io.IOException;
import java.io.InputStream;
public class VideoTypeDetector {
public static String detect(InputStream inputStream) {
try {
byte[] bytes = new byte[8];
int numBytesRead = inputStream.read(bytes, 0, bytes.length);
if (numBytesRead == bytes.length) {
if (bytes[0] == 0x52 && bytes[1] == 0x49 && bytes[2] == 0x46 && bytes[3] == 0x46) {
// AVI
return "avi";
} else if (bytes[0] == 0x00 && bytes[1] == 0x00 && bytes[2] == 0x00 && bytes[3] == 0x18 &&
bytes[4] == 0x66 && bytes[5] == 0x74 && bytes[6] == 0x79 && bytes[7] == 0x70) {
// MP4
return "mp4";
} else if (bytes[0] == 0x1A && bytes[1] == 0x45 && bytes[2] == 0xDF && bytes[3] == 0xA3) {
// MKV
return "mkv";
} else if (bytes[0] == 0x30 && bytes[1] == 0x26 && bytes[2] == 0xB2 && bytes[3] == 0x75 &&
bytes[4] == 0x8E && bytes[5] == 0x66 && bytes[6] == 0xCF && bytes[7] == 0x11) {
// WMV
return "wmv";
}
}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
}
```
该方法接受一个InputStream参数,从中读取文件开头的8个字节,并判断文件类型。如果无法识别文件类型,则返回null。使用该方法可以方便地根据InputStream获取视频文件的类型。
阅读全文
相关推荐
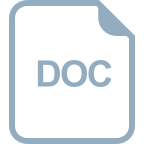
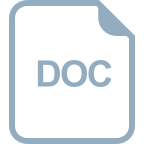
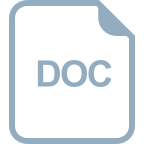
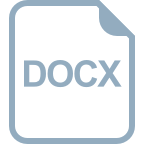
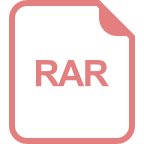
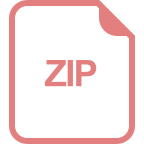
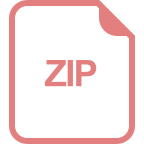
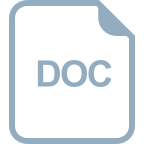
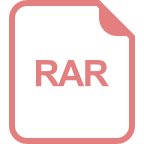
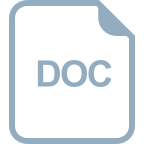
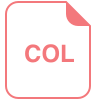
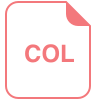
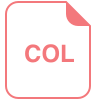
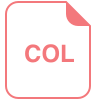




