java.util.Arrays
时间: 2024-05-02 22:21:52 浏览: 12
The java.util.Arrays class is a utility class in Java that provides various methods for working with arrays. It contains methods for sorting arrays, searching arrays, comparing arrays, and filling arrays with values.
Some of the commonly used methods of java.util.Arrays class are:
- sort(): sorts the specified array in ascending order
- binarySearch(): searches the specified array for the specified value using binary search algorithm
- toString(): returns a string representation of the contents of the specified array
- equals(): compares two arrays for equality
- fill(): assigns the specified value to each element of the specified array
Example usage:
```
int[] arr = {3, 1, 4, 1, 5, 9, 2, 6, 5};
Arrays.sort(arr); // sorts the array in ascending order
System.out.println(Arrays.toString(arr)); // prints [1, 1, 2, 3, 4, 5, 5, 6, 9]
int index = Arrays.binarySearch(arr, 5); // searches for the value 5 in the array
System.out.println(index); // prints 4
int[] arr2 = {1, 2, 3};
boolean equal = Arrays.equals(arr, arr2); // compares the two arrays for equality
System.out.println(equal); // prints false
int[] arr3 = new int[5];
Arrays.fill(arr3, 42); // fills the array with the value 42
System.out.println(Arrays.toString(arr3)); // prints [42, 42, 42, 42, 42]
```
相关推荐
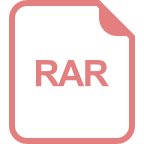














