写一个控制OV5640闪光灯的linux驱动
时间: 2024-02-27 08:57:37 浏览: 204
控制OV5640闪光灯的Linux驱动可以分为以下几个步骤:
1. 打开设备
2. 配置设备
3. 设置闪光灯
4. 关闭设备
以下是一个简单的示例代码:
```
#include <linux/module.h>
#include <linux/i2c.h>
#define OV5640_DEVICE_ADDR 0x3C
static struct i2c_client *ov5640_client;
static int ov5640_open(struct inode *inode, struct file *file)
{
// 打开设备
return 0;
}
static int ov5640_release(struct inode *inode, struct file *file)
{
// 关闭设备
return 0;
}
static long ov5640_ioctl(struct file *file, unsigned int cmd, unsigned long arg)
{
switch (cmd) {
case SET_FLASH_ON:
// 设置闪光灯
break;
case SET_FLASH_OFF:
// 设置闪光灯
break;
default:
return -EINVAL;
}
return 0;
}
static const struct file_operations ov5640_fops = {
.owner = THIS_MODULE,
.open = ov5640_open,
.release = ov5640_release,
.unlocked_ioctl = ov5640_ioctl,
};
static int ov5640_probe(struct i2c_client *client, const struct i2c_device_id *id)
{
// 配置设备
return 0;
}
static int ov5640_remove(struct i2c_client *client)
{
return 0;
}
static const struct i2c_device_id ov5640_id[] = {
{ "ov5640", 0 },
{ }
};
MODULE_DEVICE_TABLE(i2c, ov5640_id);
static struct i2c_driver ov5640_driver = {
.driver = {
.name = "ov5640",
},
.probe = ov5640_probe,
.remove = ov5640_remove,
.id_table = ov5640_id,
};
static int __init ov5640_init(void)
{
return i2c_add_driver(&ov5640_driver);
}
static void __exit ov5640_exit(void)
{
i2c_del_driver(&ov5640_driver);
}
module_init(ov5640_init);
module_exit(ov5640_exit);
MODULE_LICENSE("GPL");
```
在这个示例中,`ov5640_open()` 和 `ov5640_release()` 函数分别用于打开和关闭设备。`ov5640_ioctl()` 函数用于设置闪光灯,根据传入的命令码执行不同的操作。`ov5640_probe()` 函数用于配置设备,例如设置I2C通信地址等。`ov5640_remove()` 函数用于卸载驱动。
在驱动加载时,`ov5640_init()` 函数会注册I2C驱动,而在驱动卸载时,`ov5640_exit()` 函数会注销I2C驱动。
需要注意的是,这只是一个简单的示例代码,实际的驱动可能需要更多的配置和控制操作。同时,这个驱动也需要配合相应的用户空间应用程序使用。
阅读全文
相关推荐











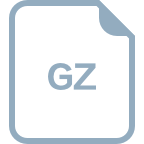