c++ redis hash to struct
时间: 2023-08-30 14:10:00 浏览: 114
To convert a Redis hash to a C++ struct, you can follow these steps:
1. Retrieve the hash values from Redis using a Redis client library in C++. For example, you can use the hiredis library or the cpp_redis library.
2. Create a C++ struct that represents the data structure you want to store in.
3. Iterate over the hash values and assign them to the corresponding struct members.
Here's an example:
```cpp
#include <iostream>
#include <cpp_redis/cpp_redis>
// Define the struct representing your data
struct MyStruct {
std::string field1;
int field2;
double field3;
};
int main() {
// Connect to Redis
cpp_redis::client client;
client.connect("127.0.0.1", 6379);
// Get the hash values from Redis
std::vector<std::string> hashValues;
client.hgetall("myhash", [&](cpp_redis::reply& reply) {
if (reply.is_array()) {
for (auto& value : reply.as_array()) {
if (value.is_string()) {
hashValues.push_back(value.as_string());
}
}
}
});
client.sync_commit();
// Convert the hash values to struct
MyStruct myStruct;
if (hashValues.size() >= 3) {
myStruct.field1 = hashValues[0];
myStruct.field2 = std::stoi(hashValues[1]);
myStruct.field3 = std::stod(hashValues[2]);
}
// Print the struct members
std::cout << "field1: " << myStruct.field1 << std::endl;
std::cout << "field2: " << myStruct.field2 << std::endl;
std::cout << "field3: " << myStruct.field3 << std::endl;
return 0;
}
```
In this example, we connect to Redis, retrieve the hash values using `hgetall`, and convert them to the `MyStruct` struct. Finally, we print the values of the struct members. Make sure to include the necessary headers and link against the Redis client library you choose to use.
阅读全文
相关推荐
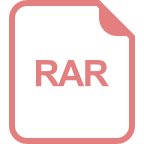
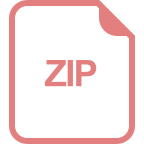
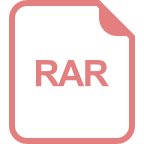
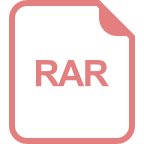
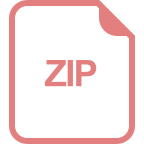
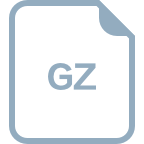
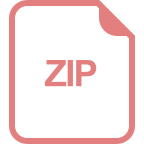
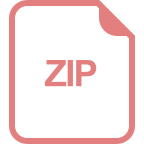
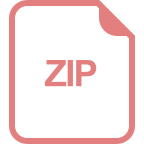
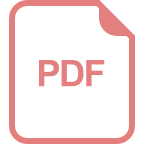
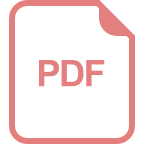
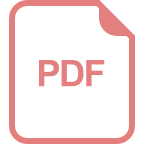
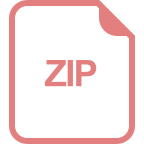
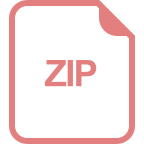
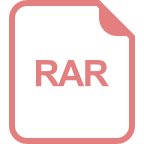
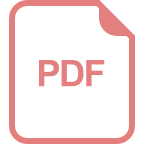