java文件下载后删除文件代码实现
时间: 2024-09-06 18:03:32 浏览: 41
在Java中,你可以使用`java.io.File`类配合`java.nio.file.Files`来实现文件下载并下载完成后立即删除文件的操作。这里是一个简单的示例:
```java
import java.io.*;
import java.net.URL;
import java.nio.file.Files;
import java.nio.file.Path;
public class FileDownloadDelete {
public static void main(String[] args) throws IOException {
// URL of the file you want to download
String url = "http://example.com/file-to-download.zip";
try (InputStream in = new URL(url).openStream();
FileOutputStream fos = new FileOutputStream("downloaded_file.zip")) {
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
fos.flush();
System.out.println("File downloaded and saved as downloaded_file.zip");
} finally {
// Delete the file after download is complete
Path filePath = Paths.get("downloaded_file.zip");
if (Files.exists(filePath)) {
Files.deleteIfExists(filePath);
System.out.println("File deleted: downloaded_file.zip");
} else {
System.out.println("File not found for deletion.");
}
}
}
}
```
这个代码首先从指定URL读取数据到内存缓冲区,然后写入到本地文件。当所有数据都写入完成后,尝试删除下载后的文件。
阅读全文
相关推荐
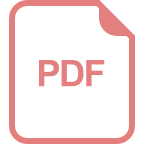
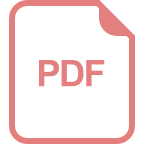
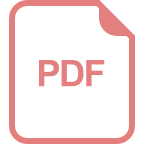
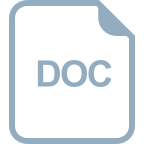
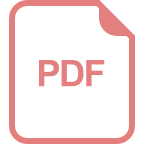
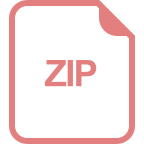
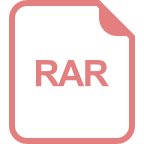
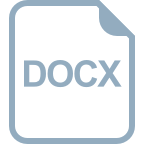
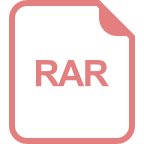
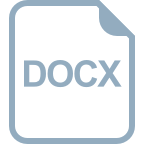
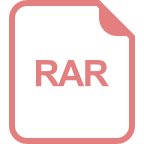
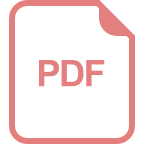
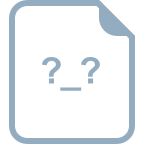
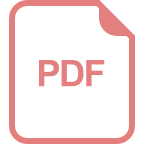
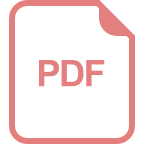
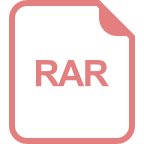
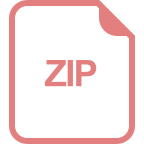