用栈进行输入十进制的数转化为二进制的数用C写出来用顺序表
时间: 2024-09-27 11:17:56 浏览: 42
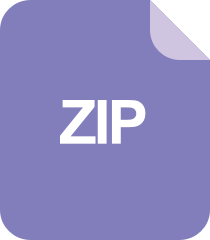
顺序栈、链栈将10进制转为2、8、16进制源码

将十进制整数转换为二进制可以利用栈的数据结构,因为栈的特点是后进先出(LIFO),适合处理按位取余的过程。以下是使用顺序表(数组)作为栈来实现这个过程的简单C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义栈结构
typedef struct {
int* stack;
int top; // 栈顶指针
int capacity; // 栈容量
} Stack;
// 初始化栈
Stack* init_stack(int capacity) {
Stack* s = (Stack*)malloc(sizeof(Stack));
s->stack = (int*)malloc(capacity * sizeof(int));
s->top = -1;
s->capacity = capacity;
return s;
}
// 入栈操作
void push(Stack* s, int value) {
if (s->top == s->capacity - 1) {
printf("Stack overflow.\n");
return;
}
s->stack[++s->top] = value;
}
// 出栈操作
int pop(Stack* s) {
if (s->top == -1) {
printf("Stack underflow.\n");
return -1;
}
return s->stack[s->top--];
}
// 转化函数
void decimal_to_binary(int num, Stack* stack) {
while (num > 0) {
push(stack, num % 2);
num /= 2;
}
}
// 打印栈内容
void print_stack(Stack* s) {
for (int i = s->top; i >= 0; i--) {
printf("%d", s->stack[i]);
}
}
int main() {
int input_num;
printf("Enter a decimal number: ");
scanf("%d", &input_num);
Stack* binary_stack = init_stack(32); // 保证足够大的栈容量防止溢出
decimal_to_binary(input_num, binary_stack);
printf("Binary representation: ");
print_stack(binary_stack);
free(binary_stack->stack);
free(binary_stack);
return 0;
}
```
在这个程序中,我们首先初始化一个栈,然后通过`decimal_to_binary`函数逐位将十进制数转换成二进制并入栈。最后打印出栈的内容即得到二进制表示。
阅读全文
相关推荐
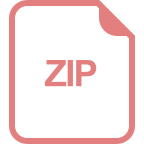
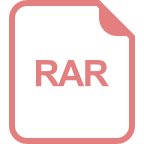


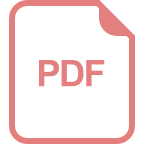
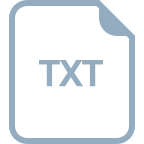
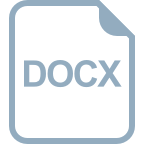
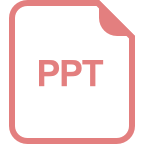
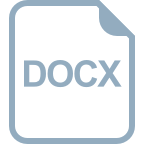
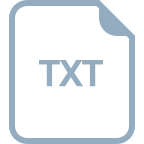
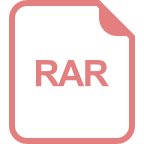
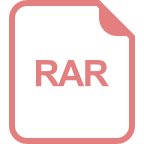
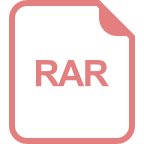
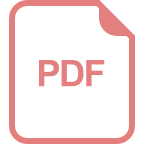
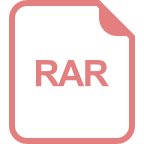
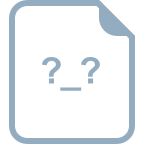
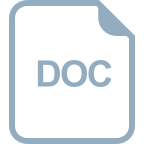
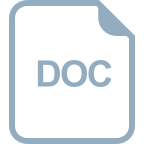