ECC B163 C代码
时间: 2024-09-30 20:15:43 浏览: 27
ECC (Error Correcting Code) B163 是一种椭圆曲线密码算法,它属于椭圆曲线加密标准的一部分,特别用于公钥加密系统。B163是一个特定的椭圆曲线,其中的参数B163意味着它定义在一个有限域上,这个域是由模p(通常是素数)构造的,而163就是这个素数。
C代码涉及到ECC B163,通常会包含一些库函数,如生成随机点、计算点的加法和乘法、密钥对生成、消息的数字签名以及公钥解密等操作。C语言中的实现可能会依赖于第三方库,比如 OpenSSL 或者内部自定义的数学库。
例如,要在C代码中工作,你可能会看到这样的片段:
```c
#include <openssl/ec.h>
EC_KEY *ec_key_new_by_curve_name(NID_secp163r1); // 使用B163曲线名创建新的密钥
BIGNUM *pub_key = ecdsa_sign_recover_message(ec_key, msg, sig_len, NULL, &raw_sig);
int ecdsa_verify(ec_key, msg, raw_sig);
// ...其他ECC相关的操作...
相关问题
ECC B163 python 代码
ECC B163是一种椭圆曲线密码算法,它属于椭圆曲线加密标准(Elliptic Curve Cryptography, ECDH或ECDSA)的一部分,常用于轻量级安全通信场景。Python中可以使用一些库来支持ECC操作,如`cryptography`库。
下面是一个简单的例子,展示如何使用`cryptography`库在Python中处理ECC B163:
```python
from cryptography.hazmat.primitives.asymmetric import ec
from cryptography.hazmat.primitives import serialization
from cryptography.hazmat.primitives import hashes
# 加载ECC B163曲线
curve = ec.SECP163K1()
# 创建私钥和公钥对象
private_key = ec.generate_private_key(curve)
public_key = private_key.public_key()
# 将公钥转换为PEM格式字符串(通常用于传输)
public_pem = public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo)
# 如果需要,也可以将私钥序列化存储
private_pem = private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.PKCS8,
encryption_algorithm=serialization.NoEncryption())
# 对消息进行数字签名
message = b"Hello, world!"
signature = private_key.sign(message, ec.ECDSA(hashes.SHA256()))
# 验证签名
try:
public_key.verify(signature, message, ec.ECDSA(hashes.SHA256()))
print("Signature verified.")
except ValueError:
print("Invalid signature.")
ecc加密c语言源代码
ecc加密算法是一种非对称加密算法,其中涉及到了椭圆曲线运算。以下是一个简单的C语言源代码示例,用于演示ecc加密过程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
#include <math.h>
// 定义椭圆曲线的参数
typedef struct {
int a;
int b;
int p;
} EllipticCurve;
// 定义椭圆曲线上的点
typedef struct {
int x;
int y;
} Point;
// 定义加密函数
Point ecc_encrypt(Point G, int k, EllipticCurve curve) {
Point Q;
Q.x = G.x;
Q.y = G.y;
for (int i = 1; i < k; i++) {
Q = ecc_add(Q, G, curve);
}
return Q;
}
// 定义点加法函数
Point ecc_add(Point P, Point Q, EllipticCurve curve) {
Point R;
// 对于两个点相等的情况,使用点加倍运算
if (P.x == Q.x && P.y == Q.y) {
return ecc_double(P, curve);
}
// 计算斜率
int m;
if (P.x != Q.x) {
m = ((Q.y - P.y) * mod_inverse(Q.x - P.x, curve.p)) % curve.p;
} else {
m = ((3 * pow(P.x, 2) + curve.a) * mod_inverse(2 * P.y, curve.p)) % curve.p;
}
// 计算新点的坐标
R.x = (pow(m, 2) - P.x - Q.x + curve.p) % curve.p;
R.y = (m * (P.x - R.x) - P.y + curve.p) % curve.p;
return R;
}
// 定义点加倍函数
Point ecc_double(Point P, EllipticCurve curve) {
Point R;
// 计算斜率
int m = ((3 * pow(P.x, 2) + curve.a) * mod_inverse(2 * P.y, curve.p)) % curve.p;
// 计算新点的坐标
R.x = (pow(m, 2) - 2 * P.x + curve.p) % curve.p;
R.y = (m * (P.x - R.x) - P.y + curve.p) % curve.p;
return R;
}
// 定义求模逆的函数
int mod_inverse(int a, int m) {
int t0 = 0;
int t1 = 1;
int r0 = m;
int r1 = a;
while (r1 != 0) {
int q = r0 / r1;
int temp = r1;
r1 = r0 - q * r1;
r0 = temp;
temp = t1;
t1 = t0 - q * t1;
t0 = temp;
}
if (t0 < 0) {
t0 += m;
}
return t0;
}
int main() {
// 创建椭圆曲线实例
EllipticCurve curve;
curve.a = 2;
curve.b = 2;
curve.p = 17;
// 创建基点实例
Point G;
G.x = 5;
G.y = 1;
// 选择一个私钥
int private_key = 6;
// 使用椭圆曲线加密
Point encrypted_point = ecc_encrypt(G, private_key, curve);
printf("加密后的点坐标:(%d, %d)\n", encrypted_point.x, encrypted_point.y);
return 0;
}
```
以上代码演示了ecc加密的基本过程。其中,定义了椭圆曲线参数、点坐标和加密函数等相关结构和函数。在`main`函数中,首先定义了椭圆曲线的参数和基点的坐标,然后选择了一个私钥。最后,调用`ecc_encrypt`函数对基点进行加密,并打印加密后的点坐标。
阅读全文
相关推荐
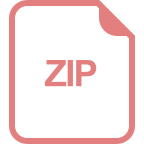
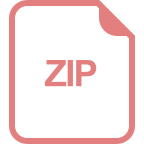
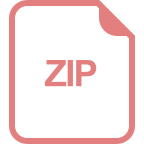

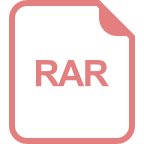
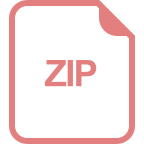
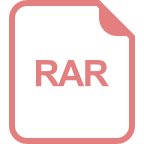

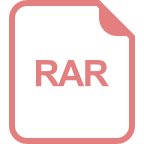
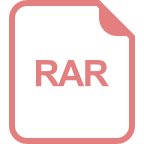
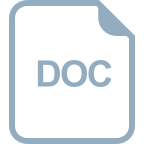
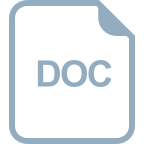
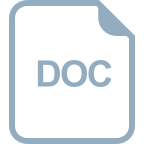



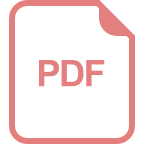