编写程序实现字符串的基本运算: (1)求串的长度、两串连接、串比较、子串匹配; (2)用库函数直接实现上一步的字符串操作;(使用C++)
时间: 2024-12-11 09:38:45 浏览: 4
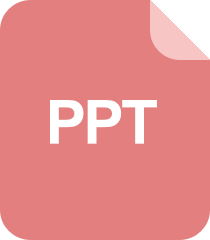
串的基本运算-第4章 字符串 串
在C++中,我们可以使用内置的标准库`<string>`来进行字符串的基本运算。这里是一些示例代码:
1. **计算字符串长度**:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
int length = str.length(); // 使用length()函数获取长度
std::cout << "String length: " << length << std::endl;
return 0;
}
```
2. **两串连接**:
```cpp
std::string concatenation(const std::string& s1, const std::string& s2) {
return s1 + s2; // 直接使用加法操作符将两个字符串连接
}
int main() {
std::string str1 = "Hello";
std::string str2 = ", World!";
std::string combined = concatenation(str1, str2);
std::cout << "Combined string: " << combined << std::endl;
return 0;
}
```
3. **串比较**:
```cpp
bool compareStrings(const std::string& s1, const std::string& s2) {
return s1 == s2; // 使用等于运算符比较字符串内容
}
int main() {
std::string str1 = "Hello";
std::string str2 = "Hello";
bool result = compareStrings(str1, str2);
std::cout << "Strings are equal: " << (result ? "Yes" : "No") << std::endl;
return 0;
}
```
4. **子串匹配**:
```cpp
bool findSubstring(const std::string& mainStr, const std::string& subStr) {
size_t found = mainStr.find(subStr);
return found != std::string::npos;
}
int main() {
std::string mainStr = "Hello, World!";
std::string substr = "World";
if (findSubstring(mainStr, substr)) {
std::cout << "Substring found: Yes" << std::endl;
} else {
std::cout << "Substring found: No" << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
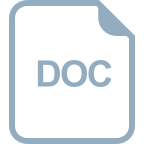
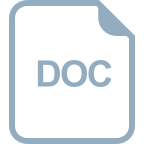

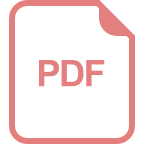
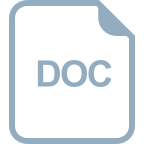
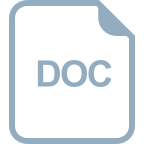
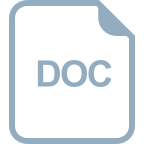
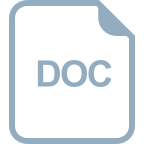
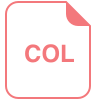
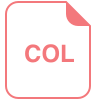
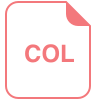
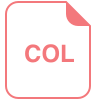
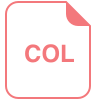
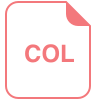
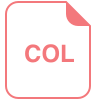

