如何计算给定字符串中’a’字符的出现次数?用c++
时间: 2024-12-11 11:36:37 浏览: 7
在C++中,你可以使用循环结构和计数器来计算给定字符串中'a'字符的出现次数。这里是一个简单的示例:
```cpp
#include <iostream>
#include <string>
int countA(const std::string& str) {
int count = 0;
for (char c : str) { // 遍历字符串中的每个字符
if (c == 'a') { // 如果字符是'a'
count++; // 计数器加一
}
}
return count; // 返回'a'字符的计数
}
int main() {
std::string input = "Hello, there are many a's in this string!";
int result = countA(input);
std::cout << "The number of 'a' characters is: " << result << std::endl;
return 0;
}
```
在这个程序中,`countA`函数接收一个字符串作为输入,然后通过遍历每一个字符并检查是否为'a'来计算其出现次数。
相关问题
如何计算给定字符串中一个字符的出现次数?用c++
在C++中,计算一个字符串中特定字符的出现次数可以使用循环和条件语句来完成。以下是一个简单的示例:
```cpp
#include <iostream>
#include <string>
int countCharacter(const std::string& str, char targetChar) {
int count = 0;
for (char c : str) {
if (c == targetChar) {
count++;
}
}
return count;
}
int main() {
std::string inputString = "Hello, World!";
char searchChar = 'o';
int occurrences = countCharacter(inputString, searchChar);
std::cout << "字符 '" << searchChar << "' 在字符串中出现了 " << occurrences << " 次.\n";
return 0;
}
```
在这个例子中,`countCharacter`函数接受一个字符串和一个目标字符作为参数,然后遍历整个字符串,如果遇到的目标字符与当前字符匹配,计数器就增加。最后返回这个计数值。
如果你想让用户输入字符串和字符,可以稍微修改main函数,比如从命令行读取或者用cin获取用户输入。
用C++编一查找给定字符串程序,要求输出给定字符串在文件中的出现的行数,第一个字符在此行中的位置。应区分给定字符串本身构成一个字和作为另外一个字的子串两种情况
这是一个查找给定字符串在文件中出现的行数和位置的 C 程序。程序应该首先读取文件,然后搜索给定字符串。如果找到了给定字符串,则应输出行号和字符串在该行的第一个字符的位置。
建议的程序框架如下:
```
#include <stdio.h>
#include <string.h>
int main(int argc, char *argv[]) {
// 首先检查命令行参数是否正确
if (argc != 3) {
printf("Usage: search string filename\n");
return 1;
}
// 然后打开文件
FILE *fp = fopen(argv[2], "r");
if (fp == NULL) {
printf("Error: unable to open file %s\n", argv[2]);
return 1;
}
// 读取文件的每一行,并检查是否包含给定字符串
char line[1024];
int lineNumber = 0;
while (fgets(line, sizeof(line), fp) != NULL) {
lineNumber++;
// 检查字符串是否是该行的子串
if (strstr(line, argv[1]) != NULL) {
// 如果找到了,输出行号和第一个字符的位置
printf("Line %d: %s", lineNumber, line);
}
}
// 关闭文件
fclose(fp);
return 0;
}
```
请注意,这是一个简单的程序框架,您可能需要对其进行修改以满足您的具体需求。例如,您可能希望在输出中显示字符串在行中的实际位置,或者您希望区分给
阅读全文
相关推荐
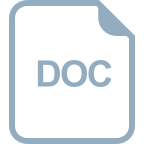
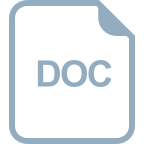
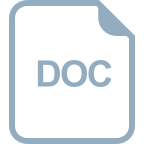













