Linux系统用c语言一段程序,访问pcie设备的io空间
时间: 2024-11-06 08:21:50 浏览: 23
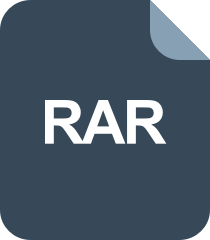
2048小游戏基于LINUX操作系统使用c语言实现
在Linux系统中,C语言可以使用内核API(如Linux PCI设备驱动程序框架)来访问PCI Express (PCIe) 设备的I/O空间。以下是一个简单的概述:
1. 包含必要的头文件:首先需要包含`<linux/pci.h>` 来获取PCI设备操作相关的宏和函数。
```c
#include <linux/pci.h>
```
2. 打开PCI设备:通过`pci_find_device()`、`pci_get_device()` 和 `pci_enable_device()` 函数找到并初始化目标PCI设备。
```c
struct pci_dev *pdev;
int ret = pci_find_device(pdev_id, NULL, &pdev);
if (ret != PCI_SUCCESS) {
// 处理错误...
}
pci_enable_device(pdev);
```
3. 获取 BAR (Base Address Register) 地址:PCI设备通常有一个或多个内存映射区域(Memory Mapped I/O, MMIO),可以通过`pci_resource_start(pdev, i)`和`pci_resource_len(pdev, i)`分别获取每个BAR的起始地址和长度。
```c
u64 bar_addr = pci_resource_start(pdev, PCI_BASE_ADDRESS_0);
size_t bar_size = pci_resource_len(pdev, PCI_BASE_ADDRESS_0);
```
4. 访问IO空间:你可以像访问普通内存一样,通过地址偏移读写数据。注意要用`ioread8`, `ioread16`, `ioread32`, `ioread64`等函数读取,用`iowrite8`, `iowrite16`, `iowrite32`, `iowrite64`等函数写入。
```c
union reg_val {
u8 byte;
u16 word;
u32 dword;
u64 qword;
};
reg_val value;
value.qword = ioread64(bar_addr + some_offset); // 读取
iorewrite64(value.qword, bar_addr + some_offset); // 写入
```
5. 关闭设备:使用完设备后记得关闭它。
```c
pci_disable_device(pdev);
pci_dev_put(pdev);
```
阅读全文
相关推荐
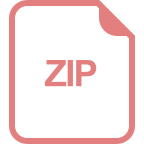
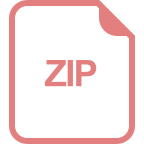
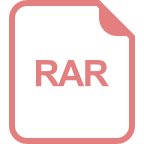
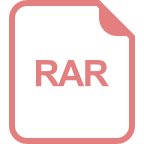
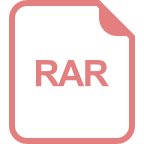
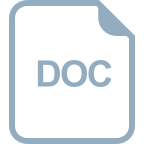
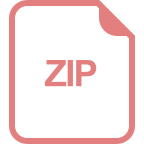
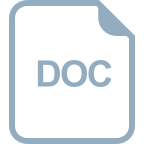
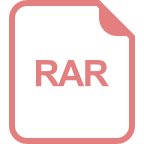
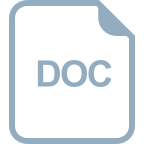
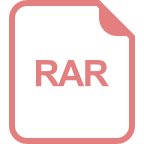
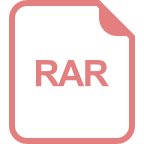
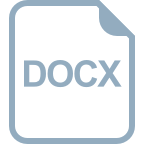
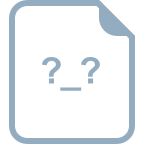