vue3+ts+uniapp微信小程序分享
时间: 2024-08-17 07:02:19 浏览: 30
在Vue3中结合TypeScript(TS)开发微信小程序,并实现分享功能,你需要做以下几步:
1. **安装依赖**:
首先确保已经安装了 Vue CLI 和 TypeScript。如果你还没有,可以使用 `vue create` 创建一个新的项目,然后添加 `@vue/cli-plugin-typescript` 插件。
```bash
npm install -D @vue/cli-plugin-typescript
```
2. **配置文件**:
更新 `vue.config.js` 添加TypeScript配置,并启用微信小程序支持。添加 `uni-app` 模板:
```js
module.exports = {
chainWebpack: (config) => {
config.resolve.symlinks(true);
// ... 其他配置
config.module
.rule('uni')
.test(/\.wxml|\.wxss|\.json$/)
.use('vue-loader')
.loader('@dcloudio/uni-template-loader');
},
transpileDependencies: ['umi', '@vue/cli-plugin-uniapp'],
};
```
3. **创建组件**:
使用TS编写你的视图组件,比如一个分享按钮:
```typescript
<template>
<button v-if="!isWechat" @click="share">分享到微信</button>
<uni-share :title="title" :desc="description" :link="link" />
</template>
<script lang="ts">
import { Component } from 'vue';
import { ShareObject } from '@dcloudio/uni-app/dist/package/share';
@Component({
components: {},
})
export default class MyComponent extends Vue {
isWechat = uni.getSystemInfoSync().platform === 'wechat'; // 判断是否微信环境
title = '我的标题';
description = '我的描述';
link = 'https://example.com'; // 分享链接
async share() {
if (this.isWechat) {
const shareOptions: ShareObject = {
title: this.title,
desc: this.description,
path: location.href, // 或者自定义路径
};
await uni.share(shareOptions);
}
}
}
</script>
```
4. **处理微信特定逻辑**:
如果在微信环境中,你可能需要处理额外的逻辑,如授权、设置默认分享行为等。这通常在生命周期钩子里完成,例如 `onLoad` 或者 `onLaunch`。
5. **测试和部署**:
完成以上步骤后,通过 `npm run serve` 启动开发服务器,然后在微信开发者工具中调试和测试分享功能。
相关推荐
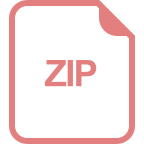
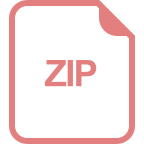
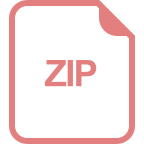














