androidstudio图片居中_android imageview图片居中技巧应用
时间: 2023-11-17 17:14:22 浏览: 35
要将Android ImageView中的图片居中,可以使用以下技巧:
1. 使用android:scaleType属性:在布局文件中,将ImageView的scaleType属性设置为centerCrop或centerInside,这将使图片在ImageView中居中显示。
2. 在代码中设置LayoutParams:在代码中,可以使用LayoutParams将ImageView的宽度和高度设置为match_parent,并将gravity属性设置为center。
3. 自定义ImageView:如果以上方法无法满足要求,可以创建一个自定义的ImageView,重写onDraw方法,并手动将图片绘制到居中位置。
下面是一个示例布局文件,使用centerCrop属性将图片居中显示:
```
<ImageView
android:id="@+id/my_image_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/my_image"
android:scaleType="centerCrop"/>
```
在代码中设置LayoutParams:
```
ImageView imageView = findViewById(R.id.my_image_view);
imageView.setLayoutParams(new ViewGroup.LayoutParams(
ViewGroup.LayoutParams.MATCH_PARENT,
ViewGroup.LayoutParams.MATCH_PARENT));
imageView.setGravity(Gravity.CENTER);
```
创建一个自定义的ImageView:
```
public class CenteredImageView extends ImageView {
public CenteredImageView(Context context) {
super(context);
}
public CenteredImageView(Context context, AttributeSet attrs) {
super(context, attrs);
}
public CenteredImageView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
}
@Override
protected void onDraw(Canvas canvas) {
Drawable drawable = getDrawable();
if (drawable == null) {
return;
}
int canvasWidth = canvas.getWidth();
int canvasHeight = canvas.getHeight();
int drawableWidth = drawable.getIntrinsicWidth();
int drawableHeight = drawable.getIntrinsicHeight();
int left = (canvasWidth - drawableWidth) / 2;
int top = (canvasHeight - drawableHeight) / 2;
drawable.setBounds(left, top, left + drawableWidth, top + drawableHeight);
drawable.draw(canvas);
}
}
```
在布局文件中使用自定义的ImageView:
```
<com.example.myapp.CenteredImageView
android:id="@+id/my_image_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/my_image"/>
```
相关推荐
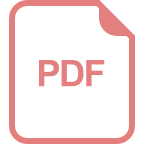
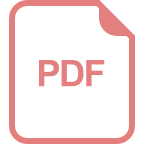
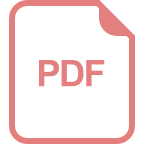






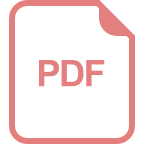
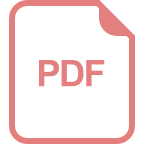
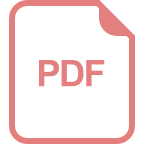
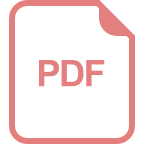
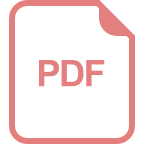
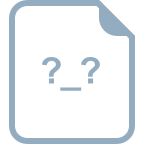
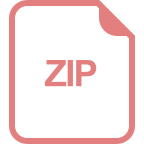