WebSockets in Ruby
时间: 2023-12-21 21:42:00 浏览: 103
WebSockets in Ruby can be implemented using various libraries and frameworks. One popular library is `faye-websocket`, which provides a simple API for working with WebSockets in Ruby.
To get started, you'll need to install the `faye-websocket` gem:
```bash
gem install faye-websocket
```
Once installed, you can use the library to create a WebSocket server or client. Here's an example of how to create a WebSocket server using `faye-websocket`:
```ruby
require 'faye/websocket'
require 'eventmachine'
EM.run {
class MyWebSocketServer
def initialize
@clients = []
end
def call(env)
if Faye::WebSocket.websocket?(env)
ws = Faye::WebSocket.new(env)
ws.on :open do |event|
@clients << ws
end
ws.on :message do |event|
@clients.each { |client| client.send(event.data) }
end
ws.on :close do |event|
@clients.delete(ws)
ws = nil
end
# Return async Rack response
ws.rack_response
else
# Handle non-WebSocket requests
[200, { 'Content-Type' => 'text/plain' }, ['Hello']]
end
end
end
# Start the WebSocket server
App = MyWebSocketServer.new
Rack::Handler::Thin.run(App, Port: 8080)
}
```
In this example, we create a WebSocket server using the `faye-websocket` library and the `EventMachine` event loop. The server listens on port 8080 and broadcasts any received messages to all connected clients.
You can also create WebSocket clients using `faye-websocket`. Here's an example of how to create a WebSocket client:
```ruby
require 'faye/websocket'
require 'eventmachine'
EM.run {
ws = Faye::WebSocket::Client.new('ws://localhost:8080')
ws.on :open do |event|
puts 'Connected to server'
ws.send('Hello server!')
end
ws.on :message do |event|
puts "Received message: #{event.data}"
end
ws.on :close do |event|
puts 'Disconnected from server'
EM.stop
end
}
```
In this example, we create a WebSocket client using the `faye-websocket` library and the `EventMachine` event loop. The client connects to a WebSocket server running at `ws://localhost:8080`, sends a message, and prints any received messages.
These examples should give you a basic understanding of how to use WebSockets in Ruby using the `faye-websocket` library. However, there are also other libraries available, such as `websocket-eventmachine-server` and `em-websocket`, which you can explore based on your specific requirements.
阅读全文
相关推荐
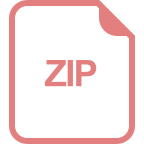
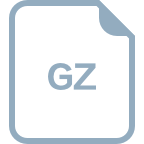
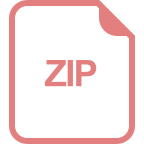
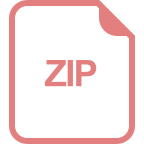
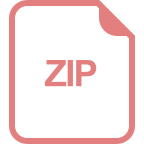
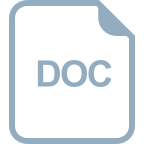
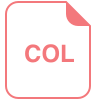
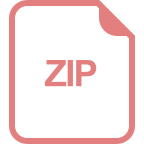
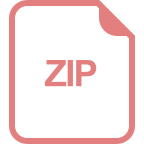
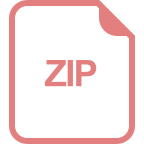
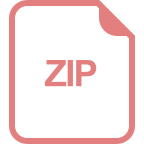
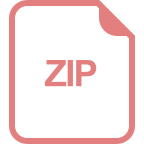