_Q = torch.gather(_Qs, 1, _action) #摇摆棍 RuntimeError: gather(): Expected dtype int64 for index
时间: 2024-02-25 22:59:06 浏览: 152
这个错误通常是因为 `gather()` 函数的第二个参数 `_action` 的数据类型不是 `torch.int64`。`gather()` 函数需要整数类型的索引来从 `_Qs` 张量中收集数据。你可以尝试将 `_action` 转换为 `torch.int64` 类型,例如:
```python
_Q = torch.gather(_Qs, 1, _action.long())
```
如果 `_action` 的数据类型已经是整数类型,你也可以尝试将其转换为 `torch.int64`,例如:
```python
_Q = torch.gather(_Qs, 1, _action.to(torch.int64))
```
如果你需要进一步帮助,请提供更多的上下文和代码。
相关问题
_Q = torch.gather(_Qs, 1, _action.to(torch.int64)) #摇摆棍 RuntimeError: Index tensor must have the same number of dimensions as input tensor
这个错误通常是因为 `_action` 张量的维度比 `_Qs` 张量的维度少了一维。在 `torch.gather()` 函数中,第二个参数 `_action` 是用于从 `_Qs` 张量中收集值的索引。这个索引张量的维度应该与 `_Qs` 张量的维度相同。如果 `_Qs` 张量的维度是 `(batch_size, num_actions)`,那么 `_action` 张量的维度应该是 `(batch_size, 1)`。
你可以尝试在使用 `torch.gather()` 函数之前给 `_action` 张量增加一维,例如:
```python
_action = _action.unsqueeze(1)
_Q = torch.gather(_Qs, 1, _action.to(torch.int64))
```
这将会在 `_action` 张量的第二个维度上增加一个维度,使其维度变为 `(batch_size, 1)`。
如果你需要进一步帮助,请提供更多的上下文和代码。
使用了_Qs = _Qs.type(torch.int64)还是出现那个问题
在使用`gather`函数时,如果出现`RuntimeError: gather(): Expected dtype int64 for index`错误,可能是因为你正在使用的是非int64类型的张量进行索引,或者索引张量中包含了非int64类型的值。以下是一些可能的解决方法:
1. 确保你的索引张量是int64类型。可以使用`.type(torch.int64)`方法将整个张量转换为int64类型。
2. 确保你的索引张量中不包含非int64类型的值。可以使用`.type(torch.int64)`方法将整个张量转换为int64类型,然后检查索引张量中是否有非int64类型的值。
3. 确保你使用的是int64类型的张量进行索引。可以使用`.type(torch.int64)`方法将整个张量转换为int64类型,然后再进行索引操作。
如果你已经尝试了以上解决方法,但仍然遇到了问题,请提供更多的代码和错误信息,以便我更好地帮助你解决问题。
阅读全文
相关推荐




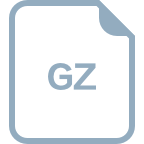











