用python画一个爱心
时间: 2023-09-25 19:06:42 浏览: 95
### 回答1:
可以使用 Python 的绘图库 matplotlib 来画一个爱心。
以下是一个简单的例子:
```
import matplotlib.pyplot as plt
import numpy as np
t = np.linspace(0, 2 * np.pi, 100)
x = 16 * np.sin(t) ** 3
y = 13 * np.cos(t) - 5 * np.cos(2 * t) - 2 * np.cos(3 * t) - np.cos(4 * t)
plt.plot(x, y)
plt.show()
```
这段代码会生成一个爱心的图形。您也可以通过调整参数来控制图形的大小、形状和颜色等。
### 回答2:
可以使用Python的turtle模块来画一个爱心。
首先,需要导入turtle模块:
```python
import turtle
```
接下来,需要设置画布大小和画笔速度:
```python
turtle.setup(800, 600)
turtle.speed(5)
```
然后,定义一个函数来画爱心:
```python
def draw_heart():
turtle.fillcolor("red")
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
turtle.circle(-90, 200)
turtle.left(120)
turtle.circle(-90, 200)
turtle.forward(224)
turtle.end_fill()
```
最后,调用函数来画出爱心:
```python
draw_heart()
```
完整的代码如下:
```python
import turtle
turtle.setup(800, 600)
turtle.speed(5)
def draw_heart():
turtle.fillcolor("red")
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
turtle.circle(-90, 200)
turtle.left(120)
turtle.circle(-90, 200)
turtle.forward(224)
turtle.end_fill()
draw_heart()
turtle.done()
```
当运行这段代码时,会弹出一个窗口显示爱心图案。可以通过调整设置画布的大小和画笔的速度来改变爱心的大小和绘制速度。
### 回答3:
在Python中,我们可以使用turtle库来绘制一个爱心形状。首先需要导入turtle库,并创建一个turtle对象,然后使用turtle对象的方法来控制绘制。下面是一个绘制爱心的示例代码:
```python
import turtle
# 创建一个turtle对象
pen = turtle.Turtle()
# 设置画笔颜色和粗细
pen.color("red")
pen.width(3)
# 将画笔移动到合适的起始位置
pen.up() # 抬起画笔
pen.goto(0, -100) # 移动到起始点
pen.down() # 放下画笔
# 绘制爱心形状
pen.begin_fill() # 开始填充
pen.left(140) # 向左旋转140度
pen.forward(224) # 向前移动224像素
pen.circle(-90, 200) # 绘制一个半径为90像素的贝塞尔曲线
pen.left(120) # 向左旋转120度
pen.circle(-90, 200) # 绘制另一个贝塞尔曲线
pen.forward(224) # 向前移动224像素
pen.end_fill() # 结束填充
# 隐藏turtle对象
pen.hideturtle()
# 显示绘制窗口
turtle.done()
```
运行以上代码,将会弹出一个窗口展示绘制的爱心形状。可以根据需要调整爱心的大小、位置或颜色等参数来实现更多样式的爱心图案。
相关推荐




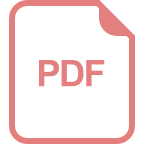
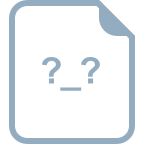
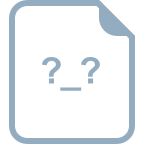