c语言switch里面能嵌套if吗
时间: 2024-10-20 18:17:38 浏览: 25
在 C 语言中,switch 语句本身是不能直接嵌套另一个 if 语句的。switch 结构用于根据不同的条件执行不同的代码块,它的每个 case 后面都跟着一个表达式,程序会根据这个表达式的值匹配到相应的 case 子句并执行其后的代码。
然而,你可以通过在 switch 语句内部使用 continue 或 break 来间接模拟类似 if 的结构。比如,在某个 case 中处理完后,可以使用 break 结束当前的 switch,然后在外部用 if 进行进一步的条件判断。如果需要连续检查多个条件,这时通常会倾向于使用链式 if-else 或者一系列独立的 switch 语句。
以下是示例:
```c
switch(expression) {
case value1:
// do something for value1
if (some_condition) {
// additional logic inside the same case
}
break;
case value2:
// do something for value2
if (another_condition) {
// more logic here
}
break;
default:
// default case or handle other values
}
```
相关问题
c语言switch case语句嵌套if else语句
在C语言中,我们可以在switch case语句中嵌套if else语句,以便根据更复杂的条件来执行特定的操作。例如:
```
switch (x) {
case 1:
if (y > 0) {
printf("x is 1 and y is positive\n");
} else {
printf("x is 1 but y is not positive\n");
}
break;
case 2:
printf("x is 2\n");
break;
default:
printf("x is neither 1 nor 2\n");
break;
}
```
在上面的代码中,我们首先使用switch case语句根据变量x的值来执行不同的操作。在case 1中,我们进一步使用if else语句来根据变量y的值来执行不同的操作。如果y大于0,则输出“x is 1 and y is positive”,否则输出“x is 1 but y is not positive”。在case 2中,我们只需要输出“x is 2”。在default中,我们输出“x is neither 1 nor 2”,表示x的值既不是1也不是2。
c语言switch case语句嵌套if else语句具体例子
以下是一个简单的例子,展示了C语言中switch case语句嵌套if else语句的用法。
```c
#include <stdio.h>
int main() {
int score = 80;
char grade;
switch (score) {
case 90:
grade = 'A';
break;
case 80:
if (score >= 85) {
grade = 'A';
} else {
grade = 'B';
}
break;
case 70:
grade = 'C';
break;
default:
grade = 'D';
break;
}
printf("Score: %d, Grade: %c\n", score, grade);
return 0;
}
```
在上述代码中,我们定义了一个名为`score`的整型变量,其值为80,并且定义了一个`grade`的字符变量。然后我们对`score`进行了switch case语句的判断,如果`score`等于90,则`grade`为字符'A';如果`score`等于80,则我们在case 80中嵌套了if else语句,判断`score`是否大于等于85,如果是,则`grade`为字符'A',否则为字符'B';如果`score`等于70,则`grade`为字符'C';如果`score`不属于前面的任何一种情况,则`grade`为字符'D'。
最后,我们使用printf函数输出了`score`和`grade`的值,结果为:
```
Score: 80, Grade: A
```
阅读全文
相关推荐
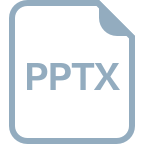
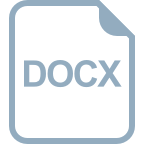
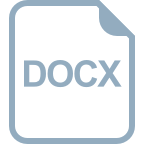


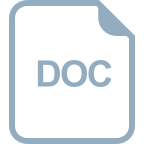
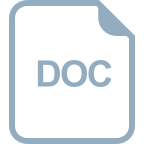
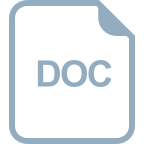
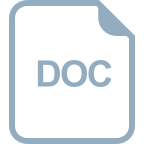
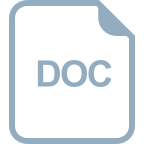





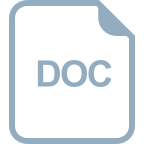
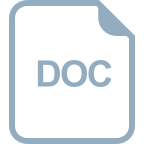