PD3.0协议实现快充需要实现哪些功能?
时间: 2023-12-25 10:05:36 浏览: 298
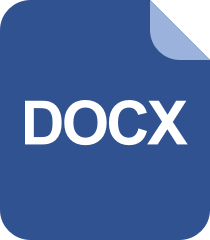
USB-PD3.0(Power Delivery)充电协议.docx

PD3.0协议是一种用于USB-C接口的快速充电协议。要实现快充功能,需要在协议的实现中添加以下功能:
1. 支持高功率输出:PD3.0协议支持高达100W的功率输出,因此快充功能需要支持高功率输出,以便快速充电。
2. 支持电压和电流的调节:快充功能需要能够动态调节输出电压和电流,以适应不同的充电设备和充电需求。
3. 支持快速识别设备:PD3.0协议支持快速识别设备的充电需求和规格,因此快充功能需要能够快速识别设备,并根据设备的充电需求进行调节。
4. 支持多种充电模式:PD3.0协议支持多种充电模式,包括恒压充电、恒流充电等,因此快充功能需要支持多种充电模式。
5. 支持安全保护:快充功能需要支持多重安全保护功能,包括过流保护、过压保护、过热保护等,以确保充电过程的安全性和稳定性。
总之,要实现PD3.0协议的快充功能,需要在协议的实现中添加多种功能,以满足不同的充电需求和规格,并确保充电过程的安全性和稳定性。
阅读全文
相关推荐
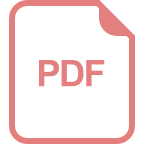
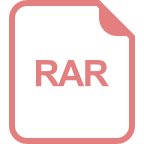


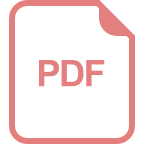
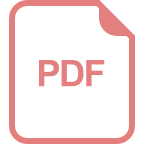
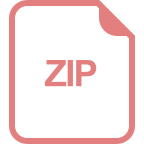
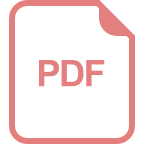
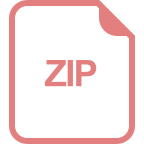
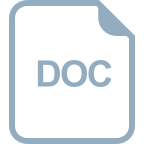
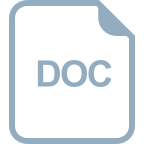
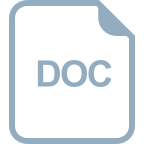

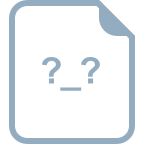
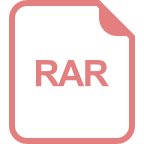
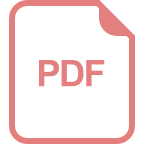
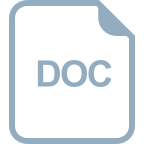
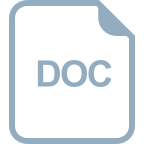