请写一个使用EtherCAT协议的数据采集程序
时间: 2023-12-02 13:05:22 浏览: 46
由于EtherCAT协议的数据采集程序需要根据具体的设备和应用场景进行开发,因此下面提供一个基本的EtherCAT数据采集程序框架,供参考:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "ecrt.h" // EtherCAT主库头文件
#define ECAT_MASTER "SOEM" // EtherCAT主库类型,可选值为SOEM、EEROS、etc.
#define ECAT_CYCLE_TIME 1000 // EtherCAT数据传输周期,单位为微秒
#define ECAT_SLAVE_NUM 1 // EtherCAT从站数量
#define ECAT_DOMAIN_NUM 1 // EtherCAT数据域数量
#define ECAT_DOMAIN_SIZE 1024 // EtherCAT数据域大小,单位为字节
// EtherCAT从站对象结构体
typedef struct {
ec_slave_config_t *config;
uint8_t *domain_ptr;
} ec_slave_t;
int main(int argc, char **argv)
{
// 初始化EtherCAT主库
if (ecrt_master_init() != 0) {
printf("Failed to initialize EtherCAT master!\n");
return -1;
}
// 获取EtherCAT主库类型
printf("EtherCAT master type: %s\n", ecrt_master_type());
// 扫描EtherCAT总线上的从站
if (ecrt_master_scan() <= 0) {
printf("No EtherCAT slaves found!\n");
return -1;
}
// 获取EtherCAT从站数量
int slave_count = ecrt_slave_count();
printf("Number of EtherCAT slaves: %d\n", slave_count);
// 分配EtherCAT从站对象数组
ec_slave_t slaves[ECAT_SLAVE_NUM];
// 配置EtherCAT从站
for (int i = 0; i < ECAT_SLAVE_NUM; i++) {
// 获取EtherCAT从站配置对象
ec_slave_config_t *config = ecrt_slave_config_ptr(i + 1);
// 检查EtherCAT从站配置对象是否为空
if (!config) {
printf("Failed to get slave config for slave %d!\n", i + 1);
return -1;
}
// 获取EtherCAT从站对象
ec_slave_t *slave = &slaves[i];
// 配置EtherCAT从站对象
slave->config = config;
slave->domain_ptr = (uint8_t *) ecrt_slave_dataptr(i + 1, 0);
// 打印EtherCAT从站信息
printf("EtherCAT slave %d: Vendor ID = 0x%X, Product ID = 0x%X\n",
i + 1, ecrt_slave_config_get_vendor_id(config),
ecrt_slave_config_get_product_code(config));
}
// 创建EtherCAT主时钟
ec_master_t *master = ecrt_master_create(ECAT_MASTER);
if (!master) {
printf("Failed to create EtherCAT master!\n");
return -1;
}
// 创建EtherCAT主时钟周期
ec_domain_t *domain = ecrt_domain_create();
if (!domain) {
printf("Failed to create EtherCAT domain!\n");
return -1;
}
// 分配EtherCAT数据域缓冲区
uint8_t *domain_ptr = (uint8_t *) malloc(ECAT_DOMAIN_SIZE);
if (!domain_ptr) {
printf("Failed to allocate EtherCAT domain buffer!\n");
return -1;
}
// 注册EtherCAT数据域
if (ecrt_domain_reg_pdo_entry_list(domain, slaves[0].config->slave,
slaves[0].config->index, slaves[0].config->vendor,
NULL, 0, NULL, ECAT_DOMAIN_SIZE) < 0) {
printf("Failed to register PDO entry list for EtherCAT domain!\n");
return -1;
}
// 设置EtherCAT数据域缓冲区
ecrt_domain_set_dataptr(domain, domain_ptr);
// 使能EtherCAT从站
if (ecrt_slave_config_pdos_enable(slaves[0].config, ECAT_DOMAIN_NUM) < 0) {
printf("Failed to enable PDOs for EtherCAT slave!\n");
return -1;
}
// 启动EtherCAT主时钟
if (ecrt_master_activate(master) != 0) {
printf("Failed to activate EtherCAT master!\n");
return -1;
}
// EtherCAT数据采集循环
while (1) {
// 循环等待EtherCAT主时钟周期
if (ecrt_master_application_time(master) >= ECAT_CYCLE_TIME) {
ecrt_master_sync_reference_clock(master);
ecrt_master_sync_slave_clocks(master);
ecrt_domain_process(domain);
// 读取EtherCAT从站数据
// TODO: Add your code here to read data from EtherCAT slaves
ecrt_domain_queue(domain);
ecrt_master_send(master);
}
}
// 停止EtherCAT主时钟
ecrt_master_deactivate(master);
// 释放EtherCAT主时钟周期
ecrt_domain_remove(domain);
// 释放EtherCAT主时钟
ecrt_master_destroy(master);
// 释放EtherCAT数据域缓冲区
free(domain_ptr);
// 关闭EtherCAT主库
ecrt_master_finalize();
return 0;
}
```
需要根据实际情况,添加读取EtherCAT从站数据的代码,例如使用ecrt_slave_receive()函数读取从站数据。同时,还需要根据具体的EtherCAT从站对象配置PDO和OD等参数,具体操作请参考EtherCAT从站设备的用户手册。
相关推荐
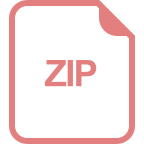
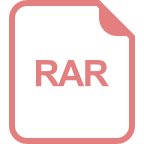










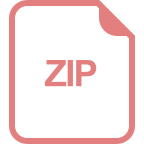
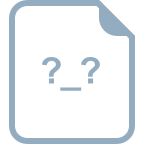
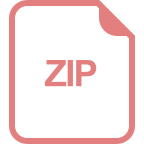