c 语言编写万年历程序,输入年、月,输出该月的日历表。输入年,输出该年的日历表。
时间: 2024-02-11 09:05:33 浏览: 419
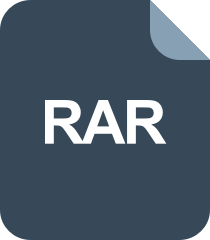
根据年份与月份,输出该月的日历
好的,这个问题需要编写一个 C 语言程序,可以通过以下步骤来实现:
1. 定义一个结构体来存储日期信息,包括年、月、日等字段。
```c
struct Date {
int year;
int month;
int day;
};
```
2. 编写一个函数来判断某年是否为闰年。闰年的判断规则是:能被4整除但不能被100整除,或者能被400整除。
```c
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
```
3. 编写一个函数来获取某个月份的天数。需要考虑到闰年的情况。
```c
int get_days_of_month(int year, int month) {
int days_of_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (month == 2 && is_leap_year(year)) {
return 29;
} else {
return days_of_month[month - 1];
}
}
```
4. 编写一个函数来获取某个月份的第一天是星期几。
```c
int get_first_day_of_month(int year, int month) {
struct Date date = {year, month, 1};
time_t time = mktime(&date);
struct tm *tm = localtime(&time);
return tm->tm_wday;
}
```
5. 编写一个函数来打印某个月份的日历表。
```c
void print_calendar(int year, int month) {
printf("%d年%d月\n", year, month);
printf("日 一 二 三 四 五 六\n");
int days_of_month = get_days_of_month(year, month);
int first_day_of_month = get_first_day_of_month(year, month);
for (int i = 0; i < first_day_of_month; i++) {
printf(" ");
}
for (int i = 1; i <= days_of_month; i++) {
printf("%2d ", i);
if ((i + first_day_of_month) % 7 == 0 || i == days_of_month) {
printf("\n");
}
}
}
```
6. 最后,编写 main 函数来接收用户输入的年份和月份,并调用 print_calendar 函数来打印日历表。
```c
int main() {
int year, month;
printf("请输入年份:");
scanf("%d", &year);
printf("请输入月份:");
scanf("%d", &month);
if (month >= 1 && month <= 12) {
print_calendar(year, month);
} else if (month == 0) {
for (int i = 1; i <= 12; i++) {
print_calendar(year, i);
printf("\n");
}
} else {
printf("无效的月份\n");
}
return 0;
}
```
这样就完成了 C 语言编写万年历程序的任务。用户可以输入年份和月份,程序会输出对应的日历表。如果用户输入的月份为0,则输出整年的日历表。
阅读全文
相关推荐





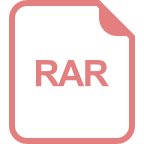
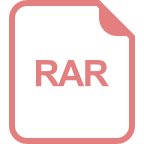
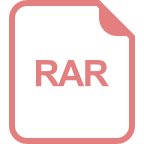
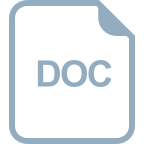


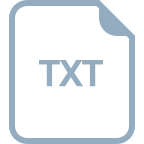
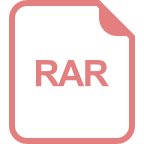
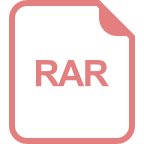